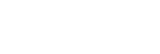 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
30 for (i = 0; i <
files->n_mesh; i++) {
72 if (
files->n_mesh == 1 &&
74 strcpy(filename,
files->meshfiles[0].filename);
80 strcpy(filename,
files->meshfiles[0].filename);
81 strtok(filename,
".");
82 strcat(filename,
".rnf");
83 if ((fp = fopen(filename,
"r")) ==
NULL) {
87 cad_filename = filename;
#define HECMW_CTRL_FTYPE_GEOFEM
int HECMW_read_abaqus_mesh(const char *filename)
void HECMW_dist_free(struct hecmwST_local_mesh *mesh)
int HECMW_log(int loglv, const char *fmt,...)
struct hecmwST_local_mesh * mesh
int HECMW_io_post_process(void)
#define HECMW_CTRL_FTYPE_HECMW_ENTIRE
#define HECMW_CTRL_FTYPE_ABAQUS
int HECMW_io_finalize(void)
#define HECMW_CTRL_FTYPE_HECMW_DIST
void HECMW_ctrl_free_meshfiles(struct hecmw_ctrl_meshfiles *meshfiles)
int HECMW_read_entire_mesh(const char *filename)
struct hecmwST_local_mesh * HECMW_get_mesh(char *name_ID)
struct hecmw_ctrl_meshfiles * HECMW_ctrl_get_meshfiles(char *name_ID)
struct hecmwST_local_mesh * HECMW_get_dist_mesh(char *fname)
struct hecmwST_local_mesh * HECMW_io_make_local_mesh(void)
int HECMW_read_geofem_mesh(const char *filename)
struct hecmw_ctrl_meshfile * meshfiles
#define HECMW_assert(cond)
#define HECMW_FILENAME_LEN
int HECMW_io_pre_process(void)
int HECMW_dist_refine(struct hecmwST_local_mesh **mesh, int refine, const char *cad_filename, const char *part_filename)