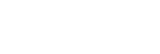 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
6 #ifndef HECMW_STRUCT_INCLUDED
7 #define HECMW_STRUCT_INCLUDED
15 #define HECMW_SECT_TYPE_SOLID 1
16 #define HECMW_SECT_TYPE_SHELL 2
17 #define HECMW_SECT_TYPE_BEAM 3
18 #define HECMW_SECT_TYPE_INTERFACE 4
21 #define HECMW_SECT_OPT_PSTRESS 0
22 #define HECMW_SECT_OPT_PSTRAIN 1
23 #define HECMW_SECT_OPT_ASYMMETRY 2
24 #define HECMW_SECT_OPT_PSTRESS_RI 10
25 #define HECMW_SECT_OPT_PSTRAIN_RI 11
26 #define HECMW_SECT_OPT_ASYMMETRY_RI 12
62 #define HECMW_AMP_TYPEDEF_TABULAR 1
65 #define HECMW_AMP_TYPETIME_STEP 1
68 #define HECMW_AMP_TYPEVAL_RELATIVE 1
69 #define HECMW_AMP_TYPEVAL_ABSOLUTE 2
85 #define HECMW_BCGRPTYPE_DISPALCEMENT 1
86 #define HECMW_BCGRPTYPE_FLUX 2
125 #define HECMW_CONTACT_TYPE_NODE_SURF 1
126 #define HECMW_CONTACT_TYPE_SURF_SURF 2
127 #define HECMW_CONTACT_TYPE_NODE_ELEM 3
143 #define HECMW_FLAG_PARTTYPE_UNKNOWN 0
144 #define HECMW_FLAG_PARTTYPE_NODEBASED 1
145 #define HECMW_FLAG_PARTTYPE_ELEMBASED 2
149 #define HECMW_FLAG_PARTCONTACT_UNKNOWN 0
150 #define HECMW_FLAG_PARTCONTACT_AGGREGATE 1
151 #define HECMW_FLAG_PARTCONTACT_DISTRIBUTE 2
152 #define HECMW_FLAG_PARTCONTACT_SIMPLE 3
struct hecmwST_refine_origin * refine_origin
int hecmw_flag_partcontact
char gridfile[HECMW_FILENAME_LEN+1]
double * elem_mat_int_val
struct hecmwST_elem_grp * elem_group
int * when_i_was_refined_elem
struct hecmwST_material * material
struct hecmwST_amplitude * amp
int * adapt_children_index
int * when_i_was_refined_node
double * node_init_val_item
struct hecmwST_section * section
int * adapt_children_item
char header[HECMW_HEADER_LEN+1]
#define HECMW_FILENAME_LEN
struct hecmwST_surf_grp * surf_group
struct hecmwST_node_grp * node_group
int * node_init_val_index
struct hecmwST_contact_pair * contact_pair
int * amp_type_definition