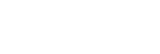 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
15 #define FSCANF_RTC_TOO_SHORT (-10001)
16 #define FSCNAF_RTC_EOF (-10002)
18 #define COLS_INT_DEF 10
19 #define COLS_DOUBLE_DEF 5
23 #define FILE_MAGIC "!HECMW-DMD-ASCII"
24 #define FILE_MAGIC_LEN (sizeof(FILE_MAGIC) - 1)
25 #define HEADER_STRING "!HECMW-DMD-ASCII version="
34 static int get_int(
int *i, FILE *fp) {
37 rtc = fscanf(fp,
"%d", i);
41 }
else if (rtc == EOF) {
52 static int get_double(
double *d, FILE *fp) {
55 rtc = fscanf(fp,
"%lf", d);
59 }
else if (rtc == EOF) {
70 static int get_string(
char *s,
int max, FILE *fp) {
73 while ((c = fgetc(fp)) != EOF && isspace(c))
79 if (ungetc(c, fp) == EOF) {
83 if (fgets(s, max, fp) ==
NULL) {
88 if (len == max - 1 && s[max - 2] !=
'\n') {
92 while (len > 0 && isspace(s[len - 1])) {
103 static int get_comm(
HECMW_Comm *i, FILE *fp) {
106 rtc = fscanf(fp,
"%d", (
int *)i);
110 }
else if (rtc == EOF) {
121 static int get_int_ary(
int *ary,
int n, FILE *fp) {
124 for (i = 0; i < n; i++) {
125 rtc = fscanf(fp,
"%d", &ary[i]);
129 }
else if (rtc == EOF) {
141 static int get_double_ary(
double *ary,
int n, FILE *fp) {
144 for (i = 0; i < n; i++) {
145 rtc = fscanf(fp,
"%lf", &ary[i]);
149 }
else if (rtc == EOF) {
161 static int get_string_ary(
char **ary,
int n, FILE *fp) {
162 #define LINEBUF_SIZE 8096
166 for (i = 0; i < n; i++) {
167 if (get_string(linebuf,
sizeof(linebuf), fp) < 0) {
184 static int is_hecmw_dist_file(FILE *fp) {
203 static int rewind_fp(FILE *fp) {
204 if (fseek(fp, 0L, SEEK_SET)) {
216 static int get_header(FILE *fp) {
295 sizeof(
char *))) ==
NULL) {
307 if (get_int(&flag_header, fp)) {
311 if (flag_header == 1) {
921 if (get_int(§->
n_sect, fp)) {
1035 if (get_int(&mat->
n_mat, fp)) {
1039 if (mat->
n_mat == 0) {
1317 if (get_int(&grp->
n_grp, fp)) {
1321 if (grp->
n_grp == 0) {
1377 if (get_int(&grp->
n_grp, fp)) {
1381 if (grp->
n_grp == 0) {
1437 if (get_int(&grp->
n_grp, fp)) {
1441 if (grp->
n_grp == 0) {
1507 if (get_int(&cpair->
n_pair, fp)) {
1511 if (cpair->
n_pair == 0) {
1526 if (get_string_ary(cpair->
name, cpair->
n_pair, fp)) {
1536 if (get_int_ary(cpair->
type, cpair->
n_pair, fp)) {
1674 if ((fp = fopen(fname,
"r")) ==
NULL) {
1680 if (!is_hecmw_dist_file(fp)) {
1685 if (rewind_fp(fp)) {
1690 if (get_header(fp)) {
1694 if (get_global_info(
mesh, fp)) {
1698 if (get_node_info(
mesh, fp)) {
1702 if (get_elem_info(
mesh, fp)) {
1706 if (get_comm_info(
mesh, fp)) {
1710 if (get_adapt_info(
mesh, fp)) {
1726 if (get_amp_info(
mesh->
amp, fp)) {
1742 if (get_refine_info(
mesh, fp)) {
1772 static int print_int(
int item, FILE *fp) {
1775 rtc = fprintf(fp,
"%d\n", item);
1787 static int print_double(
double item, FILE *fp) {
1790 rtc = fprintf(fp,
"%.16E\n", item);
1802 static int print_string(
const char *item, FILE *fp) {
1805 rtc = fprintf(fp,
"%s\n", item);
1817 static int print_comm(
HECMW_Comm item, FILE *fp) {
1821 rtc = fprintf(fp,
"%d\n", 0);
1833 static int print_int_ary(
const int *ary,
int n,
int cols, FILE *fp) {
1836 if (n <= 0)
return 0;
1838 for (i = 0; i < n; i++) {
1839 rtc = fprintf(fp,
"%d%c", ary[i], (i + 1) % cols ?
' ' :
'\n');
1846 rtc = fprintf(fp,
"\n");
1859 static int print_double_ary(
const double *ary,
int n,
int cols, FILE *fp) {
1862 if (n <= 0)
return 0;
1864 for (i = 0; i < n; i++) {
1865 rtc = fprintf(fp,
"%.16E%c", ary[i], (i + 1) % cols ?
' ' :
'\n');
1872 rtc = fprintf(fp,
"\n");
1885 static int print_string_ary(
char **ary,
int n, FILE *fp) {
1888 for (i = 0; i < n; i++) {
1889 rtc = fprintf(fp,
"%s\n", ary[i]);
1913 if (print_string(
header, fp)) {
1976 if (print_int(flag_header, fp)) {
1987 if (print_int(flag_header, fp)) {
2365 static int print_section_info(
const struct hecmwST_section *sect, FILE *fp) {
2367 if (print_int(sect->
n_sect, fp)) {
2371 if (sect->
n_sect == 0)
return 0;
2427 if (print_int(mat->
n_mat, fp)) {
2431 if (mat->
n_mat == 0)
return 0;
2488 static int print_mpc_info(
const struct hecmwST_mpc *
mpc, FILE *fp) {
2585 static int print_node_grp_info(
const struct hecmwST_node_grp *grp, FILE *fp) {
2587 if (print_int(grp->
n_grp, fp)) {
2591 if (grp->
n_grp == 0)
return 0;
2617 static int print_elem_grp_info(
const struct hecmwST_elem_grp *grp, FILE *fp) {
2619 if (print_int(grp->
n_grp, fp)) {
2623 if (grp->
n_grp == 0)
return 0;
2649 static int print_surf_grp_info(
const struct hecmwST_surf_grp *grp, FILE *fp) {
2651 if (print_int(grp->
n_grp, fp)) {
2655 if (grp->
n_grp == 0)
return 0;
2728 if (print_int(cpair->
n_pair, fp)) {
2732 if (cpair->
n_pair == 0)
return 0;
2735 if (print_string_ary(cpair->
name, cpair->
n_pair, fp)) {
2772 if (fname ==
NULL) {
2782 if ((fp = fopen(fname,
"w")) ==
NULL) {
2788 if (print_header(
mesh, fp)) {
2793 if (print_global_info(
mesh, fp)) {
2798 if (print_node_info(
mesh, fp)) {
2803 if (print_elem_info(
mesh, fp)) {
2808 if (print_comm_info(
mesh, fp)) {
2813 if (print_adapt_info(
mesh, fp)) {
2828 if (print_mpc_info(
mesh->
mpc, fp)) {
2833 if (print_amp_info(
mesh->
amp, fp)) {
2853 if (print_refine_info(
mesh, fp)) {
int hecmw_flag_partcontact
#define HECMW_malloc(size)
int HECMW_log(int loglv, const char *fmt,...)
char gridfile[HECMW_FILENAME_LEN+1]
struct hecmwST_elem_grp * elem_group
struct hecmwST_local_mesh * mesh
int HECMW_comm_get_size(void)
HECMW_Comm HECMW_comm_get_comm(void)
int HECMW_comm_get_rank(void)
int HECMW_ctrl_make_subdir(char *filename)
#define HECMW_calloc(nmemb, size)
int * when_i_was_refined_elem
struct hecmwST_material * material
struct hecmwST_amplitude * amp
int HECMW_put_dist_mesh(const struct hecmwST_local_mesh *mesh, char *fname)
#define HECMW_FLAG_PARTTYPE_ELEMBASED
int * adapt_children_index
char * HECMW_strmsg(int msgno)
int * when_i_was_refined_node
#define HECMW_FLAG_PARTTYPE_UNKNOWN
double * node_init_val_item
struct hecmwST_local_mesh * HECMW_dist_alloc()
struct hecmwST_local_mesh * HECMW_get_dist_mesh(char *fname)
int HECMW_set_error(int errorno, const char *fmt,...)
#define HECMW_FLAG_PARTTYPE_NODEBASED
struct hecmwST_section * section
#define HECMW_FLAG_PARTCONTACT_UNKNOWN
int * adapt_children_item
char header[HECMW_HEADER_LEN+1]
struct hecmwST_surf_grp * surf_group
struct hecmwST_node_grp * node_group
int * node_init_val_index
struct hecmwST_contact_pair * contact_pair
int HECMW_ctrl_is_subdir(void)
int * amp_type_definition