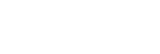 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
14 #include "parmetislib.h"
22 #define HEX_NODE_INDEX 255
23 #define HEX_FACE_INDEX 63
25 #define MAX_LINE_LEN 256
27 #define NUM_CONTROL_PARAS 9
void HECMW_dlb_memory_exit(char *str_msg)
struct _import_link_struct * next_node
char adjwgt_filename[128]
char output_filename[128]
struct _result_partition_struct Result_part
struct _control_para_struct Control_para
char adaptive_repartition[4]
struct hecmwST_result_data * new_data
void HECMW_dlb_print_exit(char *str_msg)
struct hecmwST_result_data * data
struct _adj_find_struct * next_vertex
struct hecmwST_local_mesh * new_mesh
struct hecmwST_local_mesh * mesh
struct _tmp_grp_inf Tmp_grp_inf