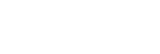 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
70 if (grp ==
NULL)
return;
72 for (i = 0; i < grp->
n_grp; i++) {
88 if (grp ==
NULL)
return;
90 for (i = 0; i < grp->
n_grp; i++) {
105 if (grp ==
NULL)
return;
107 for (i = 0; i < grp->
n_grp; i++) {
122 if (cpair ==
NULL)
return;
124 for (i = 0; i < cpair->
n_pair; i++) {
138 if (reforg ==
NULL)
return;
struct hecmwST_refine_origin * refine_origin
void HECMW_dist_free(struct hecmwST_local_mesh *mesh)
double * elem_mat_int_val
struct hecmwST_elem_grp * elem_group
struct hecmwST_local_mesh * mesh
int * when_i_was_refined_elem
void HECMW_dist_clean(struct hecmwST_local_mesh *mesh)
struct hecmwST_material * material
struct hecmwST_amplitude * amp
int HECMW_dist_init(struct hecmwST_local_mesh *mesh)
int * adapt_children_index
int * when_i_was_refined_node
double * node_init_val_item
struct hecmwST_section * section
int * adapt_children_item
struct hecmwST_surf_grp * surf_group
struct hecmwST_node_grp * node_group
int * node_init_val_index
struct hecmwST_contact_pair * contact_pair
int * amp_type_definition