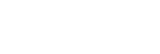 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
327 if (init_struct_global(
mesh)) {
332 if (init_struct_node(
mesh)) {
337 if (init_struct_elem(
mesh)) {
342 if (init_struct_comm(
mesh)) {
347 if (init_struct_adapt(
mesh)) {
352 if (init_struct_refine(
mesh)) {
357 if (init_struct_sect(
mesh)) {
362 if (init_struct_mat(
mesh)) {
367 if (init_struct_mpc(
mesh)) {
372 if (init_struct_amp(
mesh)) {
377 if (init_struct_node_grp(
mesh)) {
382 if (init_struct_elem_grp(
mesh)) {
387 if (init_struct_surf_grp(
mesh)) {
392 if (init_struct_contact_pair(
mesh)) {
struct hecmwST_refine_origin * refine_origin
int hecmw_flag_partcontact
char gridfile[HECMW_FILENAME_LEN+1]
double * elem_mat_int_val
struct hecmwST_elem_grp * elem_group
struct hecmwST_local_mesh * mesh
#define HECMW_calloc(nmemb, size)
int * when_i_was_refined_elem
struct hecmwST_material * material
struct hecmwST_amplitude * amp
int HECMW_dist_init(struct hecmwST_local_mesh *mesh)
int * adapt_children_index
int * when_i_was_refined_node
double * node_init_val_item
struct hecmwST_local_mesh * HECMW_dist_alloc()
int HECMW_set_error(int errorno, const char *fmt,...)
struct hecmwST_section * section
#define HECMW_assert(cond)
int * adapt_children_item
char header[HECMW_HEADER_LEN+1]
struct hecmwST_surf_grp * surf_group
struct hecmwST_node_grp * node_group
int * node_init_val_index
struct hecmwST_contact_pair * contact_pair
int * amp_type_definition