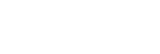 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
12 static struct hecmw_msgent msg_unknown = {-1,
"HEC-MW-UNKNOWN",
13 "Unknown message No"};
41 if (p ==
NULL) p = &msg_unknown;
51 if (dst ==
NULL)
return;
52 if (dstlen < 0)
return;
int HECMW_is_syserr(int msgno)
void HECMW_STRMSG_IF(int *msgno, char *dst, int dstlen)
void hecmw_strmsg_if__(int *msgno, char *dst, int dstlen)
int HECMW_strcpy_c2f(const char *cstr, char *fstr, int flen)
void hecmw_strmsg_if(int *msgno, char *dst, int dstlen)
char * HECMW_strmsg(int msgno)
void hecmw_strmsg_if_(int *msgno, char *dst, int dstlen)
struct hecmw_msgent hecmw_msg_table[]