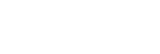 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
23 type (hecmwST_matrix),
target :: hecMAT
24 type (hecmwST_local_mesh) :: hecMESH
26 real(kind=
kreal) :: resid
27 integer(kind=kint) :: i, myrank, NDOF
28 integer(kind=kint) :: imsg = 51
37 select case(hecmat%Iarray(99))
48 if (hecmat%Iarray(97) .gt. 1) hecmat%Iarray(97)=1
53 if (hecmat%Iarray(2) .eq. 102)
then
54 if(hecmesh%PETOT.GT.1)
then
59 elseif (hecmat%Iarray(2) .eq. 104)
then
62 if(hecmesh%PETOT.GT.1)
then
72 if (hecmat%Iarray(21) > 0 .or. hecmat%Iarray(22) > 0)
then
73 write(*,
"(a,1pe12.5)")
'### Relative residual =', resid
75 if( resid >= 1.0d-8)
then
76 write(*,
"(a)")
'### Relative residual exceeded 1.0d-8---Direct Solver### '
96 type (hecmwST_local_mesh) :: hecMESH
97 type (hecmwST_matrix) :: hecMATorig
98 type (hecmwST_matrix),
pointer :: hecMAT => null()
99 integer(kind=kint) NDOF
100 if (ndof == hecmatorig%NDOF)
then
102 else if (ndof < hecmatorig%NDOF)
then
123 if (ndof /= hecmatorig%NDOF)
then
subroutine hecmw_solve(hecMESH, hecMAT)
This module provides linear equation solver interface for Pardiso.
subroutine hecmw_abort(comm)
subroutine hecmw_clone_matrix(hecMATorig, hecMAT)
subroutine hecmw_solve_iterative(hecMESH, hecMAT)
real(kind=kreal) function, public hecmw_mat_get_resid(hecMAT)
subroutine, public hecmw_solve_direct_mkl(hecMESH, hecMAT)
subroutine, public hecmw_solve_direct_parallel(hecMESH, hecMAT, ii)
subroutine, public hecmw_mat_set_flag_diverged(hecMAT, flag_diverged)
This module provides linear equation solver interface for MUMPS.
subroutine, public hecmw_solve_direct(hecMESH, hecMAT, Ifmsg)
HECMW_SOLVE_DIRECT is a program for the matrix solver.
subroutine hecmw_substitute_solver(hecMESH, hecMATorig, NDOF)
real(kind=kreal) function, public hecmw_rel_resid_l2(hecMESH, hecMAT, COMMtime)
HECMW_SOLVE_DIRECT is a program for the matrix direct solver.
integer(kind=4), parameter kreal
logical function hecmw_solve_check_zerorhs(hecMESH, hecMAT)
subroutine, public hecmw_solve_direct_mumps(hecMESH, hecMAT)
subroutine, public hecmw_mat_set_flag_converged(hecMAT, flag_converged)
integer(kind=kint) function hecmw_comm_get_rank()
subroutine, public hecmw_solve_direct_clustermkl(hecMESH, hecMAT)
integer(kind=kint) function hecmw_comm_get_comm()
subroutine hecmw_vector_contract(hecMATorig, hecMAT, NDOF)
This module provides linear equation solver interface for Cluster Pardiso.