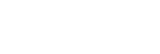 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
17 logical,
save :: INITIALIZED = .false.
18 type (sparse_matrix),
save :: spMAT
26 integer(kind=kint) :: spmat_type
27 integer(kind=kint) :: spmat_symtype
28 integer(kind=kint) :: mumps_job
29 integer(kind=kint) :: istat,
myrank
30 real(kind=
kreal) :: t1,t2,t3
37 if (initialized .and. hecmat%Iarray(98) .eq. 1)
then
41 write(*,*)
'ERROR: MUMPS returned with error', istat
48 if (.not. initialized)
then
50 if (hecmat%symmetric)
then
59 write(*,*)
'ERROR: MUMPS returned with error', istat
69 if (hecmat%Iarray(98) .eq. 1)
then
77 write(*,*)
'ERROR: MUMPS returned with error', istat
80 if (
myrank==0 .and. (spmat%iterlog > 0 .or. spmat%timelog > 0)) &
81 write(*,*)
' [MUMPS]: Analysis and Factorization completed.'
85 if (hecmat%Iarray(97) .eq. 1)
then
92 write(*,*)
'ERROR: MUMPS returned with error', istat
95 if (
myrank==0 .and. (spmat%iterlog > 0 .or. spmat%timelog > 0)) &
96 write(*,*)
' [MUMPS]: Factorization completed.'
107 write(*,*)
'ERROR: MUMPS returned with error', istat
111 if (
myrank==0 .and. (spmat%iterlog > 0 .or. spmat%timelog > 0)) &
112 write(*,*)
' [MUMPS]: Solution completed.'
115 if (
myrank==0 .and. spmat%timelog > 0)
then
116 write(*,*)
'setup time : ',t2-t1
117 write(*,*)
'solve time : ',t3-t2
subroutine, public hecmw_mat_dump_solution(hecMAT)
subroutine, public sparse_matrix_set_type(spMAT, type, symtype)
real(kind=kreal) function hecmw_wtime()
This module provides conversion routines between HEC data structure and DOF based sparse matrix struc...
subroutine, public sparse_matrix_hec_set_rhs(spMAT, hecMAT)
integer(kind=kint) myrank
PARALLEL EXECUTION.
subroutine, public sparse_matrix_finalize(spMAT)
integer(kind=kint), parameter, public sparse_matrix_symtype_spd
This module provides linear equation solver interface for MUMPS.
integer(kind=4), parameter kreal
subroutine, public sparse_matrix_hec_init_prof(spMAT, hecMAT, hecMESH)
subroutine, public sparse_matrix_hec_set_vals(spMAT, hecMAT)
subroutine, public hecmw_mumps_wrapper(spMAT, job, istat)
This module provides wrapper for parallel sparse direct solver MUMPS.
integer(kind=kint), parameter, public sparse_matrix_symtype_asym
integer(kind=kint), parameter, public sparse_matrix_type_coo
This module provides DOF based sparse matrix data structure (CSR and COO)
subroutine, public hecmw_solve_direct_mumps(hecMESH, hecMAT)
subroutine, public hecmw_mat_dump(hecMAT, hecMESH)
subroutine, public sparse_matrix_hec_get_rhs(spMAT, hecMAT)
integer(kind=kint) function hecmw_comm_get_rank()