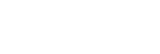 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
33 real(kind=
kreal),
save :: time_ax = 0.d0
42 subroutine hecmw_matvec (hecMESH, hecMAT, X, Y, COMMtime)
48 real(kind=
kreal),
intent(in) :: x(:)
49 real(kind=
kreal),
intent(out) :: y(:)
50 real(kind=
kreal),
intent(inout),
optional :: commtime
51 select case(hecmat%NDOF)
99 real(kind=
kreal),
intent(in) :: x(:), b(:)
100 real(kind=
kreal),
intent(out) :: r(:)
101 real(kind=
kreal),
intent(inout),
optional :: commtime
103 select case(hecmat%NDOF)
130 real(kind=
kreal),
intent(inout),
optional :: commtime
132 select case(hecmat%NDOF)
153 subroutine hecmw_tvec (hecMESH, ndof, X, Y, COMMtime)
160 integer(kind=kint),
intent(in) :: ndof
161 real(kind=
kreal),
intent(in) :: x(:)
162 real(kind=
kreal),
intent(out) :: y(:)
163 real(kind=
kreal),
intent(inout) :: commtime
179 subroutine hecmw_ttvec (hecMESH, ndof, X, Y, COMMtime)
185 integer(kind=kint),
intent(in) :: ndof
186 real(kind=
kreal),
intent(in) :: x(:)
187 real(kind=
kreal),
intent(out) :: y(:)
188 real(kind=
kreal),
intent(inout) :: commtime
209 real(kind=
kreal),
intent(in) :: x(:)
210 real(kind=
kreal),
intent(out) :: y(:), w(:)
211 real(kind=
kreal),
intent(inout) :: commtime
216 select case(hecmesh%n_dof)
258 real(kind=
kreal),
intent(inout),
optional :: commtime
260 select case(hecmesh%n_dof)
274 type (
hecmwst_matrix),
intent(inout),
target :: hecmat1, hecmat2, hecmat3
285 real(kind=
kreal),
intent(in) :: alpha
subroutine, public hecmw_matvec_unset_async
subroutine, public hecmw_matvec_11(hecMESH, hecMAT, X, Y, time_Ax, COMMtime)
subroutine, public hecmw_matvec_33(hecMESH, hecMAT, X, Y, time_Ax, COMMtime)
subroutine, public hecmw_ttvec_33(hecMESH, X, Y, COMMtime)
subroutine, public hecmw_matvec(hecMESH, hecMAT, X, Y, COMMtime)
subroutine, public hecmw_ttvec_nn(hecMESH, ndof, X, Y, COMMtime)
subroutine, public hecmw_mat_add(hecMAT1, hecMAT2, hecMAT3)
subroutine, public hecmw_matresid_33(hecMESH, hecMAT, X, B, R, time_Ax, COMMtime)
subroutine, public hecmw_matvec_clear_timer
subroutine, public hecmw_mat_multiple_nn(hecMAT, alpha)
real(kind=kreal) function, public hecmw_rel_resid_l2_66(hecMESH, hecMAT, time_Ax, COMMtime)
subroutine, public hecmw_mat_add_nn(hecMAT1, hecMAT2, hecMAT3)
subroutine, public hecmw_mat_diag_sr_nn(hecMESH, hecMAT, COMMtime)
subroutine, public hecmw_matresid(hecMESH, hecMAT, X, B, R, COMMtime)
real(kind=kreal) function, public hecmw_rel_resid_l2_44(hecMESH, hecMAT, time_Ax, COMMtime)
subroutine, public hecmw_matvec_22(hecMESH, hecMAT, X, Y, time_Ax, COMMtime)
subroutine, public hecmw_tvec_nn(hecMESH, ndof, X, Y, COMMtime)
subroutine, public hecmw_ttvec(hecMESH, ndof, X, Y, COMMtime)
subroutine, public hecmw_tvec_33(hecMESH, X, Y, COMMtime)
subroutine, public hecmw_matresid_22(hecMESH, hecMAT, X, B, R, time_Ax, COMMtime)
subroutine, public hecmw_matvec_nn(hecMESH, hecMAT, X, Y, time_Ax, COMMtime)
real(kind=kreal) function, public hecmw_rel_resid_l2(hecMESH, hecMAT, COMMtime)
subroutine, public hecmw_matvec_66(hecMESH, hecMAT, X, Y, time_Ax, COMMtime)
integer(kind=4), parameter kreal
subroutine, public hecmw_matresid_66(hecMESH, hecMAT, X, B, R, time_Ax, COMMtime)
subroutine, public hecmw_matresid_44(hecMESH, hecMAT, X, B, R, time_Ax, COMMtime)
real(kind=kreal) function, public hecmw_rel_resid_l2_33(hecMESH, hecMAT, time_Ax, COMMtime)
subroutine, public hecmw_ttmattvec(hecMESH, hecMAT, X, Y, W, COMMtime)
subroutine, public hecmw_matvec_44(hecMESH, hecMAT, X, Y, time_Ax, COMMtime)
real(kind=kreal) function, public hecmw_rel_resid_l2_nn(hecMESH, hecMAT, time_Ax, COMMtime)
real(kind=kreal) function, public hecmw_rel_resid_l2_11(hecMESH, hecMAT, time_Ax, COMMtime)
real(kind=kreal) function, public hecmw_matvec_get_timer()
subroutine, public hecmw_mat_diag_sr(hecMESH, hecMAT, COMMtime)
subroutine, public hecmw_ttmattvec_33(hecMESH, hecMAT, X, Y, W, time_Ax, COMMtime)
subroutine, public hecmw_tvec(hecMESH, ndof, X, Y, COMMtime)
real(kind=kreal) function, public hecmw_rel_resid_l2_22(hecMESH, hecMAT, time_Ax, COMMtime)
subroutine, public hecmw_ttmattvec_nn(hecMESH, hecMAT, X, Y, W, time_Ax, COMMtime)
subroutine, public hecmw_matresid_11(hecMESH, hecMAT, X, B, R, time_Ax, COMMtime)
subroutine, public hecmw_matvec_set_async(hecMAT)
subroutine, public hecmw_mat_diag_sr_33(hecMESH, hecMAT, COMMtime)
subroutine, public hecmw_matresid_nn(hecMESH, hecMAT, X, B, R, time_Ax, COMMtime)
subroutine, public hecmw_mat_multiple(hecMAT, alpha)