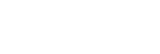 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
13 if (section ==
NULL)
return;
28 if (material ==
NULL)
return;
30 for (i = 0; i < material->
n_mat; i++) {
44 if (mpc ==
NULL)
return;
54 if (amp ==
NULL)
return;
56 for (i = 0; i < amp->
n_amp; i++) {
72 if (grp ==
NULL)
return;
74 for (i = 0; i < grp->
n_grp; i++) {
92 if (grp ==
NULL)
return;
94 for (i = 0; i < grp->
n_grp; i++) {
111 if (grp ==
NULL)
return;
113 for (i = 0; i < grp->
n_grp; i++) {
248 int mynode, pesize, i;
283 for (i = 0; i < n_component; i++) {
285 while (label_name_f[j] ==
' ') j++;
287 while (label_name_f[j] !=
' ') {
288 str_tmp[k] = label_name_f[j];
294 strcpy(label_name[i], str_tmp);
300 double *node_val_item) {
void HECMW_dlb_memory_exit(char *var)
struct hecmwST_local_mesh * new_mesh
double * elem_mat_int_val
struct hecmwST_result_data * data
struct hecmwST_elem_grp * elem_group
void hecmw_dlb_c2f_init_()
struct hecmwST_local_mesh * mesh
HECMW_Comm HECMW_comm_get_comm(void)
void dist_dlb_free_amplitude(struct hecmwST_amplitude *amp)
void hecmw_set_result_node_(int *nn_component, int *nn_dof, char *node_label, double *node_val_item)
struct hecmwST_result_data * HECMW_result_read_by_fname(char *filename)
void dist_dlb_free_mpc(struct hecmwST_mpc *mpc)
hecmw_dlb_get_result_node_(double *node_val_item)
void hecmw_dlb_f2c_init_()
void dist_dlb_free_egrp(struct hecmwST_elem_grp *grp)
int HECMW_dist_copy_c2f_init(struct hecmwST_local_mesh *local_mesh)
void dist_dlb_free_sgrp(struct hecmwST_surf_grp *grp)
void hecmw_dlb_c2f_finalize_()
void hecmw_dist_get_result_c_()
int HECMW_dist_copy_f2c_finalize(void)
int * when_i_was_refined_elem
int HECMW_dist_copy_c2f_finalize(void)
struct hecmwST_material * material
struct hecmwST_amplitude * amp
void set_label_name(int n_component, char *label_name_f, char **label_name)
struct hecmwST_result_data * new_data
hecmw_dlb_get_result_elem_(double *elem_val_item)
void hecmw_set_result_elem_(int *ne_component, int *ne_dof, char *elem_label, double *elem_val_item)
void dist_dlb_free_section(struct hecmwST_section *section)
void hecmw_dlb_f2c_finalize_()
#define HECMW_DEBUG(args)
int * when_i_was_refined_node
double * node_init_val_item
int HECMW_Comm_rank(HECMW_Comm comm, int *rank)
int HECMW_Comm_size(HECMW_Comm comm, int *size)
void dist_dlb_free_ngrp(struct hecmwST_node_grp *grp)
int HECMW_dist_copy_f2c_init(struct hecmwST_local_mesh *local_mesh)
struct hecmwST_section * section
int * adapt_children_item
void dist_dlb_free_material(struct hecmwST_material *material)
struct hecmwST_surf_grp * surf_group
struct hecmwST_node_grp * node_group
int * node_init_val_index
void HECMW_abort(HECMW_Comm comm)
int * amp_type_definition