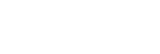 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
18 vfprintf(fp, fmt, ap);
25 vfprintf(stderr, fmt, ap);
32 static char static_buf[100];
34 if (time(&now) == (time_t)-1)
return NULL;
35 rc = strftime(static_buf,
sizeof(static_buf),
"%b %d %H:%M:%S",
37 return rc ? static_buf :
NULL;
46 if (time(&now) == (time_t)-1)
return NULL;
48 #if defined(__WIN32__) || defined(__WIN64__)
52 rc = strftime(buf, len,
"%b %d %H:%M:%S", localtime(&now));
55 if (localtime_r(&now, &result) ==
NULL)
return NULL;
56 rc = strftime(buf, len,
"%b %d %H:%M:%S", &result);
59 return rc ? buf :
NULL;
64 fprintf(stderr,
"%s:%d: Assertion `%s' failed.\n", file, line, cond_str);
78 fprintf(stderr,
"%s:%d: Assertion `%s' failed.\n", file, line, cond_str);
107 for (p = s; *p; p++) {
118 for (p = s; *p; p++) {
132 if (strlen(vmsg) > 0) {
133 HECMW_snprintf(msg + strlen(msg),
sizeof(msg) - strlen(msg),
" (%s)", vmsg);
147 return _vsnprintf(str, size, format, ap);
149 return vsnprintf(str, size, format, ap);
157 va_start(ap, format);
159 rtc = _vsnprintf(str, size, format, ap);
161 rtc = vsnprintf(str, size, format, ap);
int HECMW_check_condition_(int cond, char *cond_str, int isabort, char *file, int line)
int HECMW_snprintf(char *str, size_t size, const char *format,...)
char * HECMW_get_date(void)
void HECMW_printerr(char *fmt,...)
int HECMW_log(int loglv, const char *fmt,...)
void HECMW_print_error(void)
void HECMW_print_vmsg(int loglv, int msgno, const char *fmt, va_list ap)
char * HECMW_get_date_r(char *buf, int len)
char * HECMW_tolower(char *s)
int HECMW_comm_is_initialized(void)
void HECMW_assert_(int cond, char *cond_str, char *file, int line)
int HECMW_vsnprintf(char *str, size_t size, const char *format, va_list ap)
char * HECMW_strmsg(int msgno)
void HECMW_print_msg(int loglv, int msgno, const char *fmt,...)
char * HECMW_toupper(char *s)
char * HECMW_get_errmsg(void)
void HECMW_abort(HECMW_Comm comm)
void HECMW_fprintf(FILE *fp, char *fmt,...)