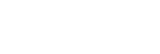 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
17 if (mat ==
NULL)
return -1;
19 if (name ==
NULL)
return -1;
22 if (strcmp(mat->
mat_name[i], name) == 0) {
28 for (i = 0; i < mat->
n_mat; i++) {
29 if (strcmp(mat->
mat_name[i], name) == 0) {
42 if (ngrp ==
NULL)
return -1;
44 if (name ==
NULL)
return -1;
46 if (i < ngrp->n_grp) {
47 if (strcmp(ngrp->
grp_name[i], name) == 0) {
53 for (i = 0; i < ngrp->
n_grp; i++) {
54 if (strcmp(ngrp->
grp_name[i], name) == 0) {
67 if (egrp ==
NULL)
return -1;
69 if (name ==
NULL)
return -1;
71 if (i < egrp->n_grp) {
72 if (strcmp(egrp->
grp_name[i], name) == 0) {
78 for (i = 0; i < egrp->
n_grp; i++) {
79 if (strcmp(egrp->
grp_name[i], name) == 0) {
92 if (sgrp ==
NULL)
return -1;
94 if (name ==
NULL)
return -1;
96 if (i < sgrp->n_grp) {
97 if (strcmp(sgrp->
grp_name[i], name) == 0) {
103 for (i = 0; i < sgrp->
n_grp; i++) {
104 if (strcmp(sgrp->
grp_name[i], name) == 0) {
118 if (i < mesh->n_node) {
140 if (i < mesh->n_elem) {
int HECMW_dist_gid2lid_node(const struct hecmwST_local_mesh *mesh, int gid)
struct hecmwST_local_mesh * mesh
int HECMW_dist_get_ngrp_id(const struct hecmwST_node_grp *ngrp, const char *name)
int HECMW_dist_get_sgrp_id(const struct hecmwST_surf_grp *sgrp, const char *name)
int HECMW_dist_gid2lid_elem(const struct hecmwST_local_mesh *mesh, int gid)
int HECMW_dist_get_egrp_id(const struct hecmwST_elem_grp *egrp, const char *name)
int HECMW_dist_get_mat_id(const struct hecmwST_material *mat, const char *name)