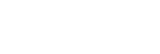 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
21 #define BINARY_DEFAULT 0
22 #define NRANK_DEFAULT 0
23 #define STRID_DEFAULT 0
24 #define ENDID_DEFAULT -1
25 #define INTID_DEFAULT 1
35 printf(
" HECMW Result File Merger\n");
36 printf(
"usage) rmerge [options] out_fileheader\n");
38 printf(
" -h : help\n");
39 printf(
" -o [type] : output file type (binary/text, default:%s)\n",
41 printf(
" -n [rank] : number of ranks (default:%d)\n",
NRANK_DEFAULT);
42 printf(
" -s [step] : start step number (default:%d)\n",
STRID_DEFAULT);
43 printf(
" -e [step] : end step number (default:%d)\n",
ENDID_DEFAULT);
44 printf(
" -i [step] : interval step number (default:%d)\n",
INTID_DEFAULT);
47 void set_fname(
int argc,
char** argv,
char* out_fheader,
int* binary,
52 for (i = 1; i < argc; i++) {
53 if (!strcmp(argv[i],
"-h")) {
56 }
else if (!strcmp(argv[i],
"-o")) {
58 fprintf(stderr,
"Error : parameter required after %s\n", argv[i]);
62 if (!strcmp(argv[i],
"binary")) {
64 }
else if (!strcmp(argv[i],
"text")) {
67 fprintf(stderr,
"Error : text or binary is required after -o\n");
70 }
else if (strcmp(argv[i],
"-n") == 0) {
72 fprintf(stderr,
"Error : parameter required after %s\n", argv[i]);
76 if (sscanf(argv[i],
"%d", nrank) != 1) {
78 "Error : parameter %s cannot be converted to number of ranks\n",
82 }
else if (strcmp(argv[i],
"-s") == 0) {
84 fprintf(stderr,
"Error : parameter required after %s\n", argv[i]);
88 if (sscanf(argv[i],
"%d",
strid) != 1) {
91 "Error : parameter %s cannot be converted to start step number\n",
95 }
else if (strcmp(argv[i],
"-e") == 0) {
97 fprintf(stderr,
"Error : parameter required after %s\n", argv[i]);
101 if (sscanf(argv[i],
"%d",
endid) != 1) {
103 "Error : parameter %s cannot be converted to end step number\n",
107 }
else if (strcmp(argv[i],
"-i") == 0) {
109 fprintf(stderr,
"Error : parameter required after %s\n", argv[i]);
113 if (sscanf(argv[i],
"%d",
intid) != 1) {
115 "Error : parameter %s cannot be converted to interval step "
129 strcpy(out_fheader, fheader);
133 int main(
int argc,
char** argv) {
139 int area_n, step_n, refine, fg_text;
153 char *ptoken, *ntoken;
160 fstr_out_log(
"out file name header is %s\n", out_fheader);
168 fprintf(stderr,
"ERROR : Cannot create global_local table.\n");
175 fprintf(stderr,
"ERROR : Cannot create global table.\n");
185 for (step =
strid; step <= step_n; step++) {
186 if ((step %
intid) != 0 && step != step_n)
continue;
199 fprintf(stderr,
"ERROR : Cannot combine result structure.\n");
213 "fstrRES", step, nrank, 0, &fg_text)) ==
NULL)
216 strcpy(buff, fileheader);
218 ptoken = strtok(buff,
"/");
219 ntoken = strtok(
NULL,
"/");
221 strcat(dirname, ptoken);
222 strcat(dirname,
"/");
224 ntoken = strtok(
NULL,
"/");
226 sprintf(out_fname,
"%s%s.%d", dirname, out_fheader, step);
233 glt->
elem_n, header, comment);
236 glt->
elem_n, header, comment);
239 fprintf(stderr,
"ERROR : Cannot open/write file %s\n", out_fname);
int fstr_get_step_n(char *name_ID, int nrank)
Check the number of steps (check for the existence of files)
char * HECMW_result_get_comment(char *buff)
char * HECMW_ctrl_get_result_fileheader_sub(char *name_ID, int istep, int n_rank, int i_rank, int *fg_text)
char * HECMW_result_get_header(char *buff)
struct hecmwST_local_mesh ** fstr_get_all_local_mesh(char *name_ID, int nrank, int *area_number, int *refine)
Read all distributed meshes.
struct hecmwST_local_mesh * mesh
void fstr_free_result(fstr_res_info **res, int area_n)
void fstr_out_log(const char *fmt,...)
Log output.
Utility for reading and processing results computed in parallel.
void fstr_free_glmesh(struct hecmwST_local_mesh *mesh)
Delete a single region mesh.
int HECMW_get_error(char **errmsg)
int HECMW_result_io_txt_write_ST_by_fname(char *filename, struct hecmwST_result_data *result, int n_node, int n_elem, char *header, char *comment)
void fstr_set_log_fp(FILE *log_fp)
Set file pointer for log output.
int HECMW_init(int *argc, char ***argv)
int HECMW_result_io_bin_write_ST_by_fname(char *filename, struct hecmwST_result_data *result, int n_node, int n_elem, char *header, char *comment)
fstr_glt * fstr_create_glt(struct hecmwST_local_mesh **mesh, int area_n)
Create table for global ID, local ID and belonging area records fstr_glt.
struct hecmwST_local_mesh * fstr_create_glmesh(fstr_glt *glt)
Create a single region mesh.
Table for global ID, local ID and belonging area records.
void fstr_free_glt(fstr_glt *glt)
Delete fstr_glt.
fstr_res_info ** fstr_get_all_result(char *name_ID, int step, int area_n, int refine, int nrank)
Read all area data of step.
int HECMW_result_init(struct hecmwST_local_mesh *hecMESH, int i_step, char *header, char *comment)
void HECMW_result_free(struct hecmwST_result_data *result)
struct hecmwST_result_data * fstr_all_result(fstr_glt *glt, fstr_res_info **res, int refine)
Combine data in all areas of the step.
#define HECMW_FILENAME_LEN
void set_fname(int argc, char **argv, char *out_fheader, int *binary, int *nrank, int *strid, int *endid, int *intid)
int main(int argc, char **argv)
void fstr_free_mesh(struct hecmwST_local_mesh **mesh, int area_n)
Delete mesh.
char * HECMW_ctrl_get_result_fileheader(char *name_ID, int istep, int *fg_text)