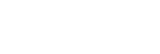 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
17 static int alloc_struct(
void) {
110 if (alloc_struct()) {
void hecmw_put_mesh_if_(char *name_ID, int *err, int len)
void hecmw_put_mesh_if__(char *name_ID, int *err, int len)
void hecmw_put_mesh_init_if__(int *err)
void HECMW_dist_free(struct hecmwST_local_mesh *mesh)
#define HECMW_malloc(size)
struct hecmwST_elem_grp * elem_group
void hecmw_put_mesh_init_if_(int *err)
void HECMW_PUT_MESH_FINALIZE_IF(int *err)
void hecmw_put_mesh_if(char *name_ID, int *err, int len)
void hecmw_put_mesh_finalize_if_(int *err)
int HECMW_dist_copy_f2c_finalize(void)
void HECMW_PUT_MESH_INIT_IF(int *err)
void HECMW_PUT_MESH_IF(char *name_ID, int *err, int len)
struct hecmwST_material * material
struct hecmwST_amplitude * amp
void hecmw_put_mesh_finalize_if(int *err)
void hecmw_put_mesh_init_if(int *err)
void hecmw_put_mesh_finalize_if__(int *err)
char * HECMW_strcpy_f2c_r(const char *fstr, int flen, char *buf, int bufsize)
int HECMW_set_error(int errorno, const char *fmt,...)
int HECMW_dist_copy_f2c_init(struct hecmwST_local_mesh *local_mesh)
struct hecmwST_section * section
struct hecmwST_surf_grp * surf_group
struct hecmwST_node_grp * node_group
struct hecmwST_contact_pair * contact_pair
int HECMW_put_mesh(struct hecmwST_local_mesh *mesh, char *name_ID)