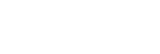 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
25 real(kind=
kreal) :: time_precond = 0.d0
33 integer(kind=kint) :: sym
51 write (*,
'(/a )')
'#### HEC-MW-SOLVER-E-1001'
52 write (*,
'( a/)')
' inconsistent solver/preconditioning'
85 real(kind=
kreal),
intent(inout) :: r(:)
86 real(kind=
kreal),
intent(inout) :: z(:), zp(:)
87 real(kind=
kreal),
intent(inout) :: commtime
88 integer(kind=kint ) :: i, n, np, nndof, npndof
89 integer(kind=kint) :: iterpremax, iterpre
90 real(kind=
kreal) :: start_time, end_time
96 nndof = n * hecmat%NDOF
97 npndof = np * hecmat%NDOF
110 do i= nndof+1, npndof
118 do iterpre= 1, iterpremax
137 do i= 1, hecmat%N * hecmat%NDOF
140 if (iterpre.eq.iterpremax)
exit
147 time_precond = time_precond + end_time - start_time
subroutine, public hecmw_precond_setup(hecMAT, hecMESH, sym)
subroutine, public hecmw_precond_sainv_clear(NDOF)
subroutine, public hecmw_precond_ssor_clear(hecMAT)
subroutine, public hecmw_precond_ssor_setup(hecMAT)
integer(kind=kint) function, public hecmw_mat_get_iterpremax(hecMAT)
real(kind=kreal) function hecmw_wtime()
subroutine hecmw_abort(comm)
integer(kind=kint) function, public hecmw_mat_get_precond(hecMAT)
subroutine, public hecmw_precond_clear(hecMAT)
subroutine, public hecmw_precond_sainv_apply(R, ZP, NDOF)
subroutine, public hecmw_precond_diag_setup(hecMAT)
subroutine, public hecmw_precond_rif_clear(NDOF)
subroutine, public hecmw_precond_ml_clear(NDOF)
subroutine, public hecmw_matresid(hecMESH, hecMAT, X, B, R, COMMtime)
subroutine, public hecmw_precond_rif_apply(ZP, NDOF)
subroutine, public hecmw_precond_bilu_clear(NDOF)
subroutine, public hecmw_precond_sainv_setup(hecMAT)
real(kind=kreal) function, public hecmw_precond_get_timer()
integer(kind=4), parameter kreal
subroutine, public hecmw_precond_diag_clear(NDOF)
subroutine, public hecmw_precond_apply(hecMESH, hecMAT, R, Z, ZP, COMMtime)
subroutine, public hecmw_precond_bilu_setup(hecMAT)
subroutine, public hecmw_precond_ml_setup(hecMAT, hecMESH, sym)
subroutine, public hecmw_precond_bilu_apply(ZP, NDOF)
subroutine, public hecmw_precond_rif_setup(hecMAT)
subroutine, public hecmw_precond_ssor_apply(ZP, NDOF)
integer(kind=kint) function hecmw_comm_get_comm()
subroutine, public hecmw_precond_ml_apply(ZP, NDOF)
subroutine, public hecmw_precond_clear_timer
subroutine, public hecmw_precond_diag_apply(ZP, NDOF)