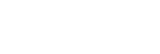 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
29 char *file,
int line) {
38 char *file,
int line) {
47 char *file,
int line) {
65 char *file,
int line) {
79 if (local_mesh->
node) {
80 for (i = 0; i < local_mesh->
n_node; i++) {
83 "%s:%d: i = %d, node[3*i] = %E, node[3*i+1] = %E, node[3*i+2] = %E",
84 file, line, i, local_mesh->
node[3 * i], local_mesh->
node[3 * i + 1],
85 local_mesh->
node[3 * i + 2]);
97 char *file,
int line) {
103 for (i = 0; i < local_mesh->
n_node; i++) {
117 char *file,
int line) {
123 for (i = 0; i < local_mesh->
n_node; i++) {
125 "%s:%d: i = %d, node_ID[2*i] = %d, node_ID[2*i+1] = %d", file,
126 line, i, local_mesh->
node_ID[2 * i],
127 local_mesh->
node_ID[2 * i + 1]);
139 char *file,
int line) {
145 for (i = 0; i < local_mesh->
n_node; i++) {
147 i, local_mesh->
node_ID[2 * i]);
159 char *file,
int line) {
165 for (i = 0; i < local_mesh->
n_node; i++) {
167 line, i, local_mesh->
node_ID[2 * i + 1]);
179 char *file,
int line) {
185 for (i = 0; i < local_mesh->
n_dof_grp; i++) {
187 "%s:%d: i = %d, node_dof_index[i] = %d, node_dof_index[i+1] = "
188 "%d, node_dof_item[i] = %d",
209 char *file,
int line) {
215 for (i = 0; i < local_mesh->
n_dof_grp; i++) {
218 "%s:%d: i = %d, node_dof_index[i] = %d, node_dof_index[i+1] = %d",
232 char *file,
int line) {
238 for (i = 0; i < local_mesh->
n_node; i++) {
240 "%s:%d: i = %d, node_init_val_index[i] = %d, "
241 "node_init_val_index[i+1] = %d",
246 j < local_mesh->node_init_val_index[i + 1]; j++) {
275 for (i = 0; i < local_mesh->
n_node; i++) {
277 "%s:%d: i = %d, node_init_val_index[i] = %d, "
278 "node_init_val_index[i+1] = %d",
306 char *file,
int line) {
315 char *file,
int line) {
324 char *file,
int line) {
332 "%s:%d: i = %d, elem_type_index[i] = %d, elem_type_index[i+1] "
333 "= %d: elem_type_item[i] = %d",
354 char *file,
int line) {
363 "%s:%d: i = %d, elem_type_index[i] = %d, elem_type_index[i+1] = %d",
377 char *file,
int line) {
383 for (i = 0; i < local_mesh->
n_elem; i++) {
397 char *file,
int line) {
403 for (i = 0; i < local_mesh->
n_elem; i++) {
406 "%s:%d: i = %d, elem_node_index[i] = %d, elem_node_index[i+1] = %d",
411 j < local_mesh->elem_node_index[i + 1]; j++) {
432 char *file,
int line) {
438 for (i = 0; i < local_mesh->
n_elem; i++) {
441 "%s:%d: i = %d, elem_node_index[i] = %d, elem_node_index[i+1] = %d",
455 char *file,
int line) {
461 for (i = 0; i < local_mesh->
n_elem; i++) {
463 "%s:%d: i = %d, elem_ID[2*i] = %d, elem_ID[2*i+1] = %d", file,
464 line, i, local_mesh->
elem_ID[2 * i],
465 local_mesh->
elem_ID[2 * i + 1]);
477 char *file,
int line) {
483 for (i = 0; i < local_mesh->
n_elem; i++) {
485 i, local_mesh->
elem_ID[2 * i]);
497 char *file,
int line) {
503 for (i = 0; i < local_mesh->
n_elem; i++) {
505 line, i, local_mesh->
elem_ID[2 * i + 1]);
517 char *file,
int line) {
523 for (i = 0; i < local_mesh->
n_elem; i++) {
537 char *file,
int line) {
557 char *file,
int line) {
563 for (i = 0; i < local_mesh->
n_elem; i++) {
577 char *file,
int line) {
586 char *file,
int line) {
592 for (i = 0; i < local_mesh->
n_elem; i++) {
594 "%s:%d: i = %d, elem_mat_ID_index[i] = %d, "
595 "elem_mat_ID_index[i+1] = %d",
600 j < local_mesh->elem_mat_ID_index[i + 1]; j++) {
621 char *file,
int line) {
627 for (i = 0; i < local_mesh->
n_elem; i++) {
629 "%s:%d: i = %d, elem_mat_ID_index[i] = %d, "
630 "elem_mat_ID_index[i+1] = %d",
649 char *file,
int line) {
658 char *file,
int line) {
678 char *file,
int line) {
686 "%s:%d: i = %d, neighbor_pe[i] = %d, import_index[i] = %d, "
687 "import_index[i+1] = %d",
691 for (j = local_mesh->
import_index[i]; j < local_mesh->import_index[i + 1];
713 char *file,
int line) {
721 "%s:%d: i = %d, neighbor_pe[i] = %d, import_index[i] = %d, "
722 "import_index[i+1] = %d",
736 char *file,
int line) {
744 "%s:%d: i = %d, neighbor_pe[i] = %d, export_index[i] = %d, "
745 "export_index[i+1] = %d",
749 for (j = local_mesh->
export_index[i]; j < local_mesh->export_index[i + 1];
771 char *file,
int line) {
779 "%s:%d: i = %d, neighbor_pe[i] = %d, export_index[i] = %d, "
780 "export_index[i+1] = %d",
794 char *file,
int line) {
802 "%s:%d: i = %d, neighbor_pe[i] = %d, shared_index[i] = %d, "
803 "shared_index[i+1] = %d",
807 for (j = local_mesh->
shared_index[i]; j < local_mesh->shared_index[i + 1];
829 char *file,
int line) {
837 "%s:%d: i = %d, neighbor_pe[i] = %d, shared_index[i] = %d, "
838 "shared_index[i+1] = %d",
870 char *file,
int line) {
878 "%s:%d: i = %d, section->sect_type[i] = %d: "
879 "section->sect_opt[i] = %d",
899 "%s:%d: i = %d, section->sect_mat_ID_index[i] = %d, "
900 "section->sect_mat_ID_index[i+1] = %d",
905 j < local_mesh->section->sect_mat_ID_index[i + 1]; j++) {
907 "%s:%d: j = %d, section->sect_mat_ID_item[j] = %d", file,
927 "%s:%d: i = %d, section->sect_I_index[i] = %d, "
928 "section->sect_I_index[i+1] = %d",
933 j < local_mesh->section->sect_I_index[i + 1]; j++) {
935 "%s:%d: j = %d, section->sect_I_item[j] = %d", file, line, j,
955 "%s:%d: i = %d, section->sect_R_index[i] = %d, "
956 "section->sect_R_index[i+1] = %d",
961 j < local_mesh->section->sect_R_index[i + 1]; j++) {
963 "%s:%d: j = %d, section->sect_R_item[j] = %E", file, line, j,
1003 char *file,
int line) {
1013 "%s:%d: i = %d, material->mat_item_index[i] = %d, "
1014 "material->mat_item_index[i+1] = %d",
1028 "%s:%d: i = %d, material->mat_name[i] = \"%s\"", file, line, i,
1044 "%s:%d: i = %d, material->mat_subitem_index[i] = %d, "
1045 "material->mat_subitem_index[i+1] = %d",
1064 "%s:%d: i = %d, material->mat_table_index[i] = %d, "
1065 "material->mat_table_index[i+1] = %d",
1070 j < local_mesh->material->mat_table_index[i + 1]; j++) {
1072 "%s:%d: j = %d, material->mat_val[j] = %E: "
1073 "material->mat_temp[j] = %E",
1127 for (i = 0; i < local_mesh->
mpc->
n_mpc; i++) {
1130 "%s:%d: i = %d, mpc->mpc_index[i] = %d, mpc->mpc_index[i+1] = %d",
1135 j < local_mesh->mpc->mpc_index[i + 1]; j++) {
1137 "%s:%d: j = %d, mpc->mpc_item[j] = %d: mpc->mpc_dof[j] = %d: "
1138 "mpc->mpc_val[j] = %E",
1192 for (i = 0; i < local_mesh->
amp->
n_amp; i++) {
1195 "%s:%d: i = %d, amp->amp_index[i] = %d, amp->amp_index[i+1] = %d",
1199 "%s:%d: i = %d, amp->amp_type_definition[i] = %d: "
1200 "amp->amp_type_time[i] = %d: amp->amp_type_value[i] = %d",
1206 j < local_mesh->amp->amp_index[i + 1]; j++) {
1208 "%s:%d: j = %d, amp->amp_val[j] = %E, amp_table[j] = %E",
1253 char *file,
int line) {
1264 char *file,
int line) {
1273 "%s:%d: i = %d, node_group->grp_index[i] = %d, "
1274 "node_group->grp_index[i+1] = %d",
1278 "%s:%d: i = %d, node_group->grp_name[i] = \"%s\"", file, line,
1282 j < local_mesh->node_group->grp_index[i + 1]; j++) {
1284 "%s:%d: j = %d, node_group->grp_item[j] = %d", file, line, j,
1316 char *file,
int line) {
1327 char *file,
int line) {
1336 "%s:%d: i = %d, elem_group->grp_index[i] = %d, "
1337 "elem_group->grp_index[i+1] = %d",
1341 "%s:%d: i = %d, elem_group->grp_name[i] = \"%s\"", file, line,
1345 j < local_mesh->elem_group->grp_index[i + 1]; j++) {
1347 "%s:%d: j = %d, elem_group->grp_item[j] = %d", file, line, j,
1379 char *file,
int line) {
1390 char *file,
int line) {
1399 "%s:%d: i = %d, surf_group->grp_index[i] = %d, "
1400 "surf_group->grp_index[i+1] = %d",
1404 "%s:%d: i = %d, surf_group->grp_name[i] = \"%s\"", file, line,
1408 j < local_mesh->surf_group->grp_index[i + 1]; j++) {
1410 "%s:%d: j = %d, surf_group->grp_item[2*i] = %d, "
1411 "surf_group->grp_item[2*i+1] = %d",
void HECMW_dbg_neighbor_pe_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_amp_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_node_dof_item_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_elem_mat_id_index_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_n_node_grp_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_elem_node_item_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_ne_internal_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_surf_group_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_import_item_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
int HECMW_log(int loglv, const char *fmt,...)
struct hecmwST_elem_grp * elem_group
void HECMW_dbg_elem_group_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_node_init_val_item_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_node_id_lid_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_elem_mat_id_item_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_n_surf_grp_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_elem_internal_list_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_n_amp_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_export_item_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_shared_item_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_elem_type_index_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_global_elem_id_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_n_elem_grp_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_n_mat_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_n_elem_mat_id_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_section_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_elem_id_domain_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_n_elem_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
struct hecmwST_material * material
void HECMW_dbg_node_group_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
struct hecmwST_amplitude * amp
void HECMW_dbg_mpc_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_nn_internal_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_node_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_n_node_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_material_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_n_node_gross_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_n_elem_type_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_elem_type_item_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_n_dof_grp_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_elem_node_index_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_nn_middle_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_node_init_val_index_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_export_index_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_global_node_id_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
double * node_init_val_item
void HECMW_dbg_node_dof_index_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_node_id_domain_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_elem_id_lid_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_section_id_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
struct hecmwST_section * section
void HECMW_dbg_n_dof_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_n_sect_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_elem_id_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
struct hecmwST_surf_grp * surf_group
struct hecmwST_node_grp * node_group
void HECMW_dbg_n_mpc_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_n_neighbor_pe_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_node_id_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
int * node_init_val_index
void HECMW_dbg_import_index_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
void HECMW_dbg_shared_index_(struct hecmwST_local_mesh *local_mesh, char *file, int line)
int * amp_type_definition
void HECMW_dbg_elem_type_(struct hecmwST_local_mesh *local_mesh, char *file, int line)