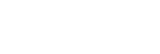 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
33 character(len=HECMW_FILENAME_LEN) ::
name_id
37 subroutine fstr_main() bind(C,NAME='fstr_main')
39 real(kind=kreal) :: t1, t2, t3
41 t1=0.0d0; t2=0.0d0; t3=0.0d0
46 myrank = hecmw_comm_get_rank()
47 nprocs = hecmw_comm_get_size()
54 if(
hecmesh%contact_pair%n_pair > 0 )
then
71 select case(
fstrpr%solution_type )
88 write(*,*)
'===================================='
89 write(*,
'(a,f10.2)')
' TOTAL TIME (sec) :', t3 - t1
90 write(*,
'(a,f10.2)')
' pre (sec) :', t2 - t1
91 write(*,
'(a,f10.2)')
' solve (sec) :', t3 - t2
92 write(*,*)
'===================================='
94 write(
imsg,*)
'===================================='
95 write(
imsg,
'(a,f10.2)')
' TOTAL TIME (sec) :', t3 - t1
96 write(
imsg,
'(a,f10.2)')
' pre (sec) :', t2 - t1
97 write(
imsg,
'(a,f10.2)')
' solve (sec) :', t3 - t2
98 write(
imsg,*)
'===================================='
107 if(
hecmesh%my_rank==0)
write(*,*)
'FrontISTR Completed !!'
119 call hecmw_nullify_matrix (
hecmat )
120 call hecmw_nullify_matrix (
conmat )
177 character(len=HECMW_FILENAME_LEN) :: s, r
178 character(len=HECMW_FILENAME_LEN) :: stafileNAME
179 character(len=HECMW_FILENAME_LEN) :: logfileNAME
180 character(len=HECMW_FILENAME_LEN) :: msgfileNAME
181 character(len=HECMW_FILENAME_LEN) :: dbgfileNAME
182 integer :: stat, flag, limit, irank
188 write( logfilename,
'(a,a)') trim(adjustl(s)),
'.log'
189 logfilename = adjustl(logfilename)
190 write( dbgfilename,
'(a,a)')
'FSTR.dbg.', trim(adjustl(s))
191 dbgfilename = adjustl(dbgfilename)
196 write( logfilename,
'(a,a,a,a,a)')
'LOG/TRUNK', trim(adjustl(r)),
'/', trim(adjustl(s)),
'.log'
197 logfilename = adjustl(logfilename)
199 if( stat /= 0 )
call fstr_setup_util_err_stop(
'### Cannot create directory' )
200 write( dbgfilename,
'(a,a,a,a,a)')
'DBG/TRUNK', trim(adjustl(r)),
'/',
'FSTR.dbg.', trim(adjustl(s))
201 dbgfilename = adjustl(dbgfilename)
203 if( stat /= 0 )
call fstr_setup_util_err_stop(
'### Cannot create directory' )
205 write( logfilename,
'(a,a,a)')
'LOG/', trim(adjustl(s)),
'.log'
206 logfilename = adjustl(logfilename)
208 if( stat /= 0 )
call fstr_setup_util_err_stop(
'### Cannot create directory' )
209 write( dbgfilename,
'(a,a,a)')
'DBG/',
'FSTR.dbg.', trim(adjustl(s))
210 dbgfilename = adjustl(dbgfilename)
212 if( stat /= 0 )
call fstr_setup_util_err_stop(
'### Cannot create directory' )
215 stafilename =
'FSTR.sta'
216 msgfilename =
'FSTR.msg'
221 open(
imsg, file=msgfilename, status=
'replace', iostat=stat)
223 call fstr_setup_util_err_stop(
'### Cannot open message file :'//msgfilename )
225 write(
imsg,*)
':========================================:'
226 write(
imsg,*)
':** BEGIN FSTR Structural Analysis **:'
227 write(
imsg,*)
':========================================:'
228 write(
imsg,*)
' Total no. of processors: ',
nprocs
232 write(
imsg,*)
' * STAGE Initialization and input **'
236 open (
ilog, file = logfilename, status =
'replace', iostat=stat )
238 call fstr_setup_util_err_stop(
'### Cannot open log file :'//logfilename )
242 open (
ista,file = stafilename, status =
'replace', iostat=stat )
243 write(
ista,
'(''####''a80)') stafilename
245 call fstr_setup_util_err_stop(
'### Cannot open status file :'//stafilename )
249 open (
idbg,file = dbgfilename, status =
'replace')
250 write(
idbg,
'(''####''a80)') dbgfilename
252 call fstr_setup_util_err_stop(
'### Cannot open debug file :'//dbgfilename )
260 character(len=HECMW_FILENAME_LEN) :: cntfileNAME
263 call hecmw_ctrl_get_control_file(
name_id, cntfilename )
277 if(
myrank == 0)
write(*,*)
'fstr_setup: OK'
278 write(
ilog,*)
'fstr_setup: OK'
299 if(
myrank == 0)
write(
imsg,*)
' *** STAGE Non Linear static analysis **'
301 if(
myrank == 0 )
write(
imsg,*)
' *** STAGE Linear static analysis **'
324 write(
imsg,*)
' *** STAGE Eigenvalue analysis **'
343 write(
imsg,*)
' *** STAGE Heat analysis **'
364 write(
imsg,*)
' *** STAGE Nonlinear dynamic analysis **'
366 write(
imsg,*)
' *** STAGE Linear dynamic analysis **'
389 write(
imsg,*)
' *** STAGE Static -> Eigen analysis **'
390 write(*,*)
' *** STAGE Static -> Eigen analysis **'
392 write(
imsg,*)
' *** Stage 1: Nonlinear static analysis **'
393 write(*,*)
' *** Stage 1: Nonlinear static analysis **'
400 write(
imsg,*)
' *** Stage 2: Eigenvalue analysis **'
402 write(*,*)
' *** Stage 2: Eigenvalue analysis **'
422 write(
imsg,*)
':========================================:'
423 write(
imsg,*)
':** END of FSTR **:'
424 write(
imsg,*)
':========================================:'
subroutine fstr_static_analysis
Master subroutine of linear/nonlinear static analysis !
integer(kind=kint), pointer iecho
FLAG for ECHO/RESULT/POST.
subroutine fstr_heat_analysis
Master subroutine of heat analysis !
This module provides functions to initialize heat analysis.
This module contains subroutines controlling dynamic calculation.
void hecmw_ctrl_is_subdir(int *flag, int *limit)
subroutine fstr_main()
Startup routine for FrontISTR.
subroutine fstr_solve_nlgeom(hecMESH, hecMAT, fstrSOLID, hecLagMAT, fstrPARAM, conMAT)
This module provides main subroutine for nonlinear calculation.
subroutine fstr_init_file
Open all files preparing calculation.
subroutine fstr_eigen_init(fstrEIG)
Initial setting of eigen ca;culation.
This module provides a function to control eigen analysis.
subroutine fstr_mat_init(hecMAT)
Initializer of structure hecmwST_matrix.
This module provide a function to ECHO for IFSTR solver.
This module provides main suboruitne for nonliear calculation.
subroutine fstr_finalize
Finalizer !
integer(kind=kint), pointer ineutral
This module provides functions to read in data from control file and do necessary preparation for fol...
integer(kind=kint) myrank
PARALLEL EXECUTION.
Package of data used by Lanczos eigenvalue solver.
integer(kind=kint), parameter kststaticeigen
type(fstr_heat), save fstrheat
subroutine heat_init_material(hecMESH, fstrHEAT)
Data for HEAT ANSLYSIS (fstrHEAT)
subroutine fstr_param_init(fstrPARAM, hecMESH)
Initializer of structure fstr_param.
This module contains functions to print out calculation settings.
type(fstr_dynamic), save fstrdynamic
character(len=hecmw_filename_len) name_id
type(hecmwst_matrix), save hecmat
type(fstr_eigen), save fstreig
subroutine fstr_init
Initializer !
subroutine fstr_nullify_fstr_dynamic(DY)
type(hecmwst_matrix), save conmat
Data for DYNAMIC ANSLYSIS (fstrDYNAMIC)
subroutine fstr_nullify_fstr_heat(H)
integer(kind=kint), parameter idbg
type(hecmwst_result_data), save fstrresult
subroutine fstr_nullify_fstr_eigen(E)
type(fstr_param), target fstrpr
GLOBAL VARIABLE INITIALIZED IN FSTR_SETUP.
subroutine fstr_setup(cntl_filename, hecMESH, fstrPARAM, fstrSOLID, fstrEIG, fstrHEAT, fstrDYNAMIC, fstrCPL, fstrFREQ)
Read in and initialize control data !
subroutine fstr_dynamic_init(fstrDYNAMIC)
Initial setting of dynamic calculation.
real(kind=kreal) dt
ANALYSIS CONTROL for NLGEOM and HEAT.
subroutine fstr_init_condition
Read in control file and do all preparation.
subroutine, public fstr_rcap_initialize(hecMESH, fstrPARAM, fstrCPL)
integer(kind=kint), parameter kststatic
type(fstr_couple), save fstrcpl
This module defines common data and basic structures for analysis.
integer(kind=kint) nprocs
subroutine hecmw2fstr_mesh_conv(hecMESH)
integer(kind=kint), pointer ivisual
void hecmw_ctrl_make_subdir(char *filename, int *err, int len)
type(hecmwst_local_mesh), save hecmesh
real(kind=kreal), pointer ref_temp
REFTEMP.
subroutine fstr_solve_eigen(hecMESH, hecMAT, fstrEIG, fstrSOLID, fstrRESULT, fstrPARAM, hecLagMAT)
solve eigenvalue probrem
Data for coupling analysis.
subroutine fstr_nullify_fstr_couple(C)
subroutine fstr_input_precheck(hecMESH, hecMAT, fstrSOLID)
fstr_input_precheck !
integer(kind=kint), parameter kstheat
integer(kind=kint), pointer iresult
subroutine fstr_solid_finalize(fstrSOLID)
Finalizer of fstr_solid.
integer(kind=kint), parameter ksteigen
subroutine fstr_nullify_fstr_param(P)
NULL POINTER SETTING TO AVOID RUNTIME ERROR.
subroutine fstr_solve_heat(hecMESH, hecMAT, fstrRESULT, fstrPARAM, fstrHEAT)
integer(kind=kint), pointer iwres
integer(kind=kint), parameter ista
HECMW to FSTR Mesh Data Converter. Converting Connectivity of Element Type 232, 342 and 352.
This module provides a function to control heat analysis.
This module provides function to check input data of IFSTR solver.
subroutine fstr_dynamic_analysis
Master subroutine of dynamic analysis !
subroutine fstr_eigen_analysis
Master subroutine of eigen analysis !
subroutine, public fstr_rcap_finalize(fstrPARAM, fstrCPL)
type(hecmwst_matrix_lagrange), save heclagmat
subroutine hecmat_init(hecMAT)
type(fstr_solid), save fstrsolid
integer(kind=kint), pointer irres
integer(kind=kint), pointer nrres
subroutine fstr_solid_init(hecMESH, fstrSOLID)
Initializer of structure fstr_solid.
subroutine fstr_nullify_fstr_solid(S)
logical paracontactflag
PARALLEL CONTACT FLAG.
subroutine fstr_echo(hecMESH)
ECHO for IFSTR solver.
subroutine fstr_static_eigen_analysis
Master subroutine of static -> eigen analysis !
subroutine heat_echo(p, hecMESH, fstrHEAT)
integer(kind=kint), dimension(100) sviarray
SOLVER CONTROL.
subroutine fstr_solve_dynamic(hecMESH, hecMAT, fstrSOLID, fstrEIG, fstrDYNAMIC, fstrRESULT, fstrPARAM, fstrCPL, fstrFREQ, hecLagMAT, conMAT)
Master subroutine for dynamic analysis.
integer(kind=kint), parameter ilog
FILE HANDLER.
integer(kind=kint), pointer nprint
real(kind=kreal), dimension(100) svrarray
subroutine fstr_heat_init(fstrHEAT)
Initial setting of heat analysis.
subroutine hecmat_finalize(hecMAT)
subroutine heat_init_amplitude(hecMESH, fstrHEAT)
type(fstr_freqanalysis), save fstrfreq
integer(kind=kint), parameter kstdynamic
integer(kind=kint), parameter imsg