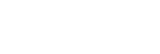 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
24 static int is_check_memleak;
28 static int auto_check = 1;
40 info = malloc(
sizeof(*info));
62 static int del_info(
void *
ptr) {
71 for (p = mainfo, i = 0; p && p->
ptr !=
ptr; p = (q = p)->
next, i++) {
92 static int change_info(
void *ptrold,
void *ptrnew,
size_t sizenew,
char *
file,
103 for (p = mainfo, i = 0; p && p->
ptr != ptrold; p = p->
next, i++) {
125 if (fp ==
NULL) fp = stdout;
128 for (p = mainfo; p; p = p->
next) {
129 fprintf(fp,
"HEC-MW memory info: %s:%d ptr=%p size=%d\n", p->
file,
142 if (mainfo ==
NULL)
return 0;
144 for (p = mainfo; p; p = p->
next) {
146 "HEC-MW memory check: "
147 "A memory leak found at %s:%d ptr=%p size=%d\n",
152 "HEC-MW memory check: "
153 "%d memory leak%s found\n",
154 n, (n > 1) ?
"s" :
"");
160 static int mark_check_memleak(
void) {
161 if (!is_check_memleak) {
162 if (atexit(check_memleak) == -1)
return -1;
163 is_check_memleak = 1;
174 "Not found allocated memory %p(%s:%d)\n",
ptr,
file,
line);
186 if (mark_check_memleak())
goto error;
201 if (mark_check_memleak())
goto error;
217 "Not found registered memory %p(%s:%d)\n",
ptr,
file,
228 "Not found registered memory %p(%s:%d)\n",
ptr,
file,
235 "Not found registered memory %p(%s:%d)\n",
ptr,
file,
242 if (mark_check_memleak())
goto error;
252 if (str ==
NULL)
goto error;
253 if (add_info(str, strlen(str) + 1,
file,
line))
goto error;
255 if (mark_check_memleak())
goto error;
int HECMW_list_meminfo(FILE *fp)
int HECMW_check_memleak(void)
void * HECMW_malloc_(size_t size, char *file, int line)
void HECMW_free_(void *ptr, char *file, int line)
void HECMW_set_autocheck_memleak(int flag)
void * HECMW_realloc_(void *ptr, size_t size, char *file, int line)
struct malloc_info * next
char * HECMW_strdup_(const char *s, char *file, int line)
long HECMW_get_memsize(void)
void HECMW_print_msg(int loglv, int msgno, const char *fmt,...)
void * HECMW_calloc_(size_t nmemb, size_t size, char *file, int line)
#define HECMW_assert(cond)