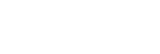 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
34 if (mesh_src ==
NULL) {
36 "HECMW_couple_make_pre_ip_list(): 'mesh_src' is NULL");
39 if (boundary_src ==
NULL) {
41 "HECMW_couple_make_pre_ip_list(): 'boundary_src' is NULL");
44 if (comm_src ==
NULL) {
46 "HECMW_couple_make_pre_ip_list(): 'comm_src' is NULL");
62 if (p->next ==
NULL)
goto error;
66 p->next = HECMW_couple_e2n_by_average(mesh_src, boundary_src);
67 if(p->next ==
NULL)
goto error;
93 if (comm_src ==
NULL) {
95 "HECMW_couple_make_main_ip_list(): 'comm_src' is NULL");
98 if (comm_dst ==
NULL) {
100 "HECMW_couple_make_main_ip_list(): 'comm_dst' is NULL");
103 if (intercomm ==
NULL) {
105 "HECMW_couple_make_main_ip_list(): 'intercomm' is NULL");
108 if (inter_tbl ==
NULL) {
110 "HECMW_couple_make_main_ip_list(): 'inter_tbl' is NULL");
114 if (mesh_src ==
NULL) {
116 "HECMW_couple_make_main_ip_list(): 'mesh_src' is NULL");
119 if (boundary_src ==
NULL) {
122 "HECMW_couple_make_main_ip_list(): 'boundary_src' is NULL");
127 if (mesh_dst ==
NULL) {
129 "HECMW_couple_make_main_ip_list(): 'mesh_dst' is NULL");
132 if (boundary_dst ==
NULL) {
135 "HECMW_couple_make_main_ip_list(): 'boundary_dst' is NULL");
138 if (mapped_point ==
NULL) {
141 "HECMW_couple_make_main_ip_list(): 'mapped_point' is NULL");
151 intercomm, boundary_src, boundary_dst,
152 mapped_point, inter_tbl);
153 if (p->next ==
NULL)
goto error;
157 intercomm, boundary_src, boundary_dst,
158 mapped_point, inter_tbl);
159 if (p->next ==
NULL)
goto error;
184 if (mesh_dst ==
NULL) {
186 "HECMW_couple_make_post_ip_list(): 'mesh_dst' is NULL");
189 if (boundary_dst ==
NULL) {
191 "HECMW_couple_make_post_ip_list(): 'boundary_dst' is NULL");
194 if (mapped_point ==
NULL) {
196 "HECMW_couple_make_post_ip_list(): 'mapped_point' is NULL");
199 if (comm_dst ==
NULL) {
201 "HECMW_couple_make_post_ip_list(): 'comm_dst' is NULL");
218 if (p->next ==
NULL)
goto error;
struct hecmw_couple_weight_list * next
struct hecmw_couple_weight_list * HECMW_couple_s2n_dist_node(const struct hecmwST_local_mesh *mesh_src, const struct hecmwST_local_mesh *mesh_dst, const struct hecmw_couple_comm *comm_src, const struct hecmw_couple_comm *comm_dst, const struct hecmw_couple_comm *intercomm, const struct hecmw_couple_boundary *boundary_src, const struct hecmw_couple_boundary *boundary_dst, const struct hecmw_couple_mapped_point *mapped_point, const struct hecmw_couple_inter_iftable *inter_tbl)
struct hecmw_couple_weight_list * HECMW_couple_s2n_average(const struct hecmwST_local_mesh *mesh, const struct hecmw_couple_boundary *boundary)
#define HECMW_COUPLE_ELEMENT_GROUP
#define HECMWCPL_E_INVALID_DATATYPE
struct hecmw_couple_weight_list * HECMW_couple_s2n_dist_surf(const struct hecmwST_local_mesh *mesh_src, const struct hecmwST_local_mesh *mesh_dst, const struct hecmw_couple_comm *comm_src, const struct hecmw_couple_comm *comm_dst, const struct hecmw_couple_comm *intercomm, const struct hecmw_couple_boundary *boundary_src, const struct hecmw_couple_boundary *boundary_dst, const struct hecmw_couple_mapped_point *mapped_point, const struct hecmw_couple_inter_iftable *inter_tbl)
struct hecmw_couple_weight_list * HECMW_couple_make_main_ip_list(const struct hecmwST_local_mesh *mesh_src, const struct hecmwST_local_mesh *mesh_dst, const struct hecmw_couple_boundary *boundary_src, const struct hecmw_couple_boundary *boundary_dst, const struct hecmw_couple_mapped_point *mapped_point, const struct hecmw_couple_comm *comm_src, const struct hecmw_couple_comm *comm_dst, const struct hecmw_couple_comm *intercomm, const struct hecmw_couple_inter_iftable *inter_tbl)
struct hecmw_couple_weight_list * HECMW_couple_n2s_average(const struct hecmwST_local_mesh *mesh, const struct hecmw_couple_boundary *boundary, const struct hecmw_couple_comm *intracomm, const struct hecmw_couple_intra_iftable *intra_tbl)
#define HECMW_COUPLE_NODE_GROUP
#define HECMW_COUPLE_SURFACE_GROUP
struct hecmw_couple_weight_list * HECMW_couple_make_pre_ip_list(const struct hecmwST_local_mesh *mesh_src, const struct hecmw_couple_boundary *boundary_src, const struct hecmw_couple_comm *comm_src, const struct hecmw_couple_intra_iftable *intra_tbl_src)
struct hecmw_couple_weight_list * HECMW_couple_make_post_ip_list(const struct hecmwST_local_mesh *mesh_dst, const struct hecmw_couple_boundary *boundary_dst, const struct hecmw_couple_mapped_point *mapped_point, const struct hecmw_couple_comm *comm_dst, const struct hecmw_couple_intra_iftable *intra_tbl_dst)
int HECMW_set_error(int errorno, const char *fmt,...)
#define HECMWCPL_E_INVALID_ARG
struct hecmw_couple_weight_list * HECMW_couple_alloc_weight_list(void)