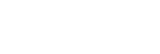 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
void hecmw_couple_startup_final_if_(int *err)
int HECMW_couple_copy_c2f_init(struct hecmw_couple_value *_couple_value)
void HECMW_COUPLE_STARTUP_INIT_IF(char *boundary_id, int *err, int len)
void HECMW_COUPLE_STARTUP_FINAL_IF(int *err)
void hecmw_couple_startup_init_if_(char *boundary_id, int *err, int len)
void hecmw_couple_startup_final_if(int *err)
char * HECMW_strcpy_f2c_r(const char *fstr, int flen, char *buf, int bufsize)
void hecmw_couple_startup_init_if__(char *boundary_id, int *err, int len)
void hecmw_couple_startup_init_if(char *boundary_id, int *err, int len)
void HECMW_couple_free_couple_value(struct hecmw_couple_value *couple_value)
struct hecmw_couple_value * HECMW_couple_startup(const char *boundary_id)
int HECMW_couple_copy_c2f_finalize(void)
void hecmw_couple_startup_final_if__(int *err)