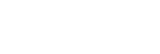 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
29 if (couple_value ==
NULL)
return;
60 if (couple_value ==
NULL || fp ==
NULL)
return;
62 fprintf(fp,
"*** Value of coupling area\n");
64 fprintf(fp,
"number of item: %d\n", couple_value->
n);
67 fprintf(fp,
"item type: NODE GROUP\n");
69 fprintf(fp,
"item type: ELEMENT GROUP\n");
71 fprintf(fp,
"item type: SURFACE GROUP\n");
73 fprintf(fp,
"item type: UNKNOWN\n");
76 fprintf(fp,
"number of DOF: %d\n", couple_value->
n_dof);
78 fprintf(fp,
"ID & value:\n");
79 for (i = 0; i < couple_value->
n; i++) {
81 fprintf(fp,
" %d %d", couple_value->
item[2 * i],
82 couple_value->
item[2 * i + 1]);
84 fprintf(fp,
" %d", couple_value->
item[i]);
87 for (j = 0; j < couple_value->
n_dof; j++) {
88 fprintf(fp,
" %lE", couple_value->
value[i * couple_value->
n_dof + j]);
97 const char *boundary_id) {
103 if (boundary_id ==
NULL) {
105 "HECMW_couple_startup(): 'boundary_id' is NULL");
115 couple_value->
n_dof = 0;
120 couple_value->
n = boundary->
node->
n;
122 if (couple_value->
n > 0) {
128 for (i = 0; i < couple_value->
n; i++) {
135 couple_value->
n = boundary->
elem->
n;
137 if (couple_value->
n > 0) {
143 for (i = 0; i < couple_value->
n; i++) {
150 couple_value->
n = boundary->
surf->
n;
152 if (couple_value->
n > 0) {
159 for (i = 0; i < couple_value->
n; i++) {
160 couple_value->
item[2 * i] = boundary->
surf->
item[2 * i];
161 couple_value->
item[2 * i + 1] = boundary->
surf->
item[2 * i + 1];
struct hecmw_couple_boundary_item * surf
struct hecmw_couple_boundary * boundary_src
#define HECMW_malloc(size)
void HECMW_couple_print_couple_value(const struct hecmw_couple_value *couple_value, FILE *fp)
#define HECMW_COUPLE_ELEMENT_GROUP
struct hecmw_couple_value * HECMW_couple_alloc_couple_value(void)
struct hecmw_couple_info * HECMW_couple_get_info(const char *boundary_id)
#define HECMW_COUPLE_NODE_GROUP
#define HECMW_COUPLE_SURFACE_GROUP
#define HECMW_COUPLE_GROUP_UNDEF
struct hecmw_couple_boundary_item * elem
struct hecmw_couple_boundary_item * node
struct hecmw_couple_comm * comm_src
int HECMW_set_error(int errorno, const char *fmt,...)
void HECMW_couple_free_couple_value(struct hecmw_couple_value *couple_value)
struct hecmw_couple_value * HECMW_couple_startup(const char *boundary_id)
#define HECMWCPL_E_INVALID_ARG
#define HECMWCPL_E_INVALID_GRPTYPE
void HECMW_couple_cleanup(struct hecmw_couple_value *couple_value)