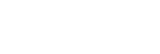 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
14 while (buf[i] !=
'\n') {
30 else if ((buf[0] ==
'!') && (buf[1] ==
'!'))
41 while ((buf[i] ==
',') || (buf[i] ==
' ') || (buf[i] ==
'=')) i++;
43 fprintf(stderr,
"No string value for %s\n", para);
45 "ERROR: HEC-MW-VIS-E0002: The control parameter format error: should "
49 while ((buf[i] !=
' ') && (buf[i] !=
',') && (buf[i] !=
'\n')) {
65 while ((buf[i] ==
',') || (buf[i] ==
' ') || (buf[i] ==
'=')) i++;
68 "ERROR: HEC-MW-VIS-E0003:The control parameter format error:No integer "
72 while ((buf[i] !=
' ') && (buf[i] !=
',') && (buf[i] !=
'\n')) {
78 if ((isdigit(para2[0]) == 0) && (para2[0] !=
'+') && (para2[0] !=
'-')) {
79 fprintf(stderr,
"ERROR: HEC-MW-VIS-E0004: %s should be integer \n", para);
93 while ((buf[i] ==
',') || (buf[i] ==
' ') || (buf[i] ==
'=')) i++;
95 fprintf(stderr,
"No integer value for %s\n", para);
99 while ((buf[i] !=
' ') && (buf[i] !=
',') && (buf[i] !=
'\n')) {
105 if ((isdigit(para2[0]) == 0) && (para2[0] !=
'+') && (para2[0] !=
'-')) {
106 fprintf(stderr,
"ERROR: HEC-MW-VIS-E0005:%s should be a real \n", para);
118 while (buf[i] ==
' ') i++;
120 fprintf(stderr,
"Please check the line %s\n", buf);
125 while ((buf[i] !=
' ') && (buf[i] !=
'=') && (buf[i] !=
',') &&
138 char para[128], para2[128];
141 while (buf[i] ==
' ') i++;
144 "ERROR: HEC-MW-DLB-E0002: The control parameter format error: should "
148 while ((buf[i] !=
' ') && (buf[i] !=
',') && (buf[i] !=
'\n')) {
154 if ((strncmp(para,
"DLB_CTRL", 8) != 0) &&
155 (strncmp(para,
"dlb_ctrl", 8) != 0)) {
192 while ((buf[i] ==
',') || (buf[i] ==
' ')) i++;
193 while (buf[i] !=
'\n') {
195 while ((buf[i] !=
' ') && (buf[i] !=
',') && (buf[i] !=
'=') &&
202 if ((strncmp(para,
"method", 6) == 0) ||
203 (strncmp(para,
"METHOD", 6) == 0)) {
205 if ((strncmp(para,
"ADAPT", 5) == 0) || (strncmp(para,
"adapt", 5) == 0))
209 while ((buf[i] ==
',') || (buf[i] ==
' ')) i++;
221 char para[128], para1[128];
225 int location, visual_method;
226 int flag, flag_surface;
231 fp = fopen(contfile,
"r");
234 parameters[i] = (
char *)calloc(128,
sizeof(
char));
235 if (parameters[i] ==
NULL) {
236 fprintf(stderr,
"There is no enough memory for parameters\n");
241 parameters[0] =
"adaptive_repartition";
243 parameters[1] =
"num_of_criteria";
245 parameters[2] =
"balance_rate";
247 parameters[3] =
"num_of_repartition";
249 parameters[4] =
"itr_rate";
251 parameters[5] =
"wgtflag";
253 parameters[6] =
"vwgt_filename";
255 parameters[7] =
"adjwgt_filename";
257 parameters[8] =
"machine_wgt";
278 while (cont_flag == 1) {
287 while (cont_flag == 1) {
291 if ((strncmp(para, parameters[i], len_para[i])) == 0) {
337 ctl_para->
machine_wgt = (
float *)calloc(pesize,
sizeof(
float));
338 for (i = 0; i < pesize; i++) {
358 if (cont_flag == 0)
break;
373 if (stat_para[1] == 0) {
380 "#### HEC-MW-DLB-E1001: num_of_criteria should be greater than 0\n");
381 fprintf(stderr,
"Please re-input a correct one\n");
384 if (stat_para[2] == 0) {
393 "#### HEC-MW-DLB-E1002: The balance rate should be >=1.0\n");
394 fprintf(stderr,
"Please input again\n");
399 if (stat_para[3] == 0) {
405 "#### HEC-MW-DLB-E1003: The num_of_repartition cannot less than 1\n");
406 fprintf(stderr,
"Please input again\n");
409 if (stat_para[4] == 0) {
413 fprintf(stderr,
"#### HEC-MW-DLB-E1004:itr_rate cannot be less than 0.0\n");
417 if (stat_para[5] == 0) {
421 fprintf(stderr,
"#### HEC-MW-DLB-E1005:wgtflag only can be in 0--3\n");
424 if (stat_para[8] == 0) {
425 ctl_para->
machine_wgt = (
float *)calloc(pesize,
sizeof(
float));
426 for (i = 0; i < pesize; i++) ctl_para->
machine_wgt[i] = 1.0 / (
float)pesize;
440 ctl_para->
balance_rate = (
float *)calloc(1,
sizeof(
float));
447 ctl_para->
machine_wgt = (
float *)calloc(pesize,
sizeof(
float));
448 for (i = 0; i < pesize; i++) ctl_para->
machine_wgt[i] = 1.0 / (
float)pesize;
#define NUM_CONTROL_PARAS
int HECMW_dlb_get_int_item(char *para, char *buf, int *start_location)
char adjwgt_filename[128]
int HECMW_dlb_get_keyword_item(char *buf, char *para)
int HECMW_dlb_get_keyword_repart(char *buf, Control_para *ctl_para)
double HECMW_dlb_get_double_item(char *para, char *buf, int *start_location)
char adaptive_repartition[4]
int HECMW_dlb_is_comment_line(char *buf)
void hecmw_dlb_set_default_control(Control_para *ctl_para, int stat_para[NUM_CONTROL_PARAS], int pesize)
void hecmw_dlb_read_control(char *contfile, Control_para *ctl_para, int stat_para[NUM_CONTROL_PARAS], int pesize)
int HECMW_dlb_is_blank_line(char *buf)
void HECMW_dlb_get_string_item(char *para, char *buf, int *start_location, char para2[128])
void HECMW_dlb_print_exit(char *var)