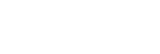 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
13 for (
int i = 0; i < 4; i++) {
14 for (
int j = 0; j < 4; j++) {
23 for (
int i = 0; i < 4; i++) {
24 for (
int j = 0; j < 4; j++) {
35 for (
int i = 0; i < 4; i++) {
36 for (
int j = 0; j < 4; j++) {
45 for (
int i = 0; i < 4; i++) {
46 for (
int j = 0; j < 4; j++) {
53 for (
int i = 0; i < 4; i++) {
54 for (
int j = 0; j < 4; j++) {
72 double s = sin(angle);
73 double c = cos(angle);
74 e[3][0] =
e[3][1] =
e[3][2] = 0.0;
144 double &Y,
double &Z) {
145 X =
e[0][0] * x +
e[0][1] * y +
e[0][2] * z +
e[0][3];
146 Y =
e[1][0] * x +
e[1][1] * y +
e[1][2] * z +
e[1][3];
147 Z =
e[2][0] * x +
e[2][1] * y +
e[2][2] * z +
e[2][3];
151 double &y,
double &z) {
158 double &y,
double &z) {
159 double R = r * cos(b);
168 for (
int i = 0; i < 4; i++) {
169 for (
int j = 0; j < 4; j++) {
172 for (
int k = 0; k < 4; k++) {
173 r += a(i, k) * b(k, j);
void cylinder2cartesian(double r, double a, double h, double &x, double &y, double &z)
void transfer(double x, double y, double z)
void convert(double x, double y, double z, double &X, double &Y, double &Z)
cconv_mat operator*(cconv_mat &a, cconv_mat &b)
void cartesian_convert(double x, double y, double z, double &X, double &Y, double &Z)
void sphere2cartesian(double r, double a, double b, double &x, double &y, double &z)
cconv_mat(coord_type t=coord_t_cartesian)
void rotate(char axis, double angle)
cconv_mat & operator=(const cconv_mat &m)
cconv_mat & operator*=(const cconv_mat &m)