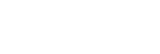 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
17 integer(kind=kint),
dimension(:) :: src
18 integer(kind=kint) :: n_data,ierr
26 real(kind=
kreal),
dimension(:) :: src
27 integer(kind=kint) :: n_data,ierr
35 integer(kind=kint) :: ierr
43 integer(kind=kint) :: ierr
44 character(len=HECMW_NAME_LEN) :: name_ID
55 integer(kind=kint) :: ierr
63 integer(kind=kint) :: ierr
64 character(len=HECMW_NAME_LEN) :: name_ID
72 integer(kind=kint) :: ierr
80 integer(kind=kint) :: ierr
81 integer(kind=kint),
dimension(:) :: dst
89 integer(kind=kint) :: ierr
90 real(kind=
kreal),
dimension(:) :: dst
subroutine hecmw_restart_write_by_name(name_ID)
subroutine hecmw_abort(comm)
subroutine hecmw_restart_write()
subroutine hecmw_restart_add_real(src, n_data)
void hecmw_restart_read_real_if(double *dst, int *err)
subroutine hecmw_restart_close()
subroutine hecmw_restart_open()
subroutine hecmw_restart_read_int(dst)
void hecmw_restart_close_if(int *err)
subroutine hecmw_restart_read_real(dst)
void hecmw_restart_read_int_if(int *dst, int *err)
subroutine hecmw_restart_add_int(src, n_data)
integer(kind=4), parameter kreal
subroutine hecmw_restart_open_by_name(name_ID)
void hecmw_restart_write_by_name_if(char *name_ID, int *err, int len)
void hecmw_restart_open_by_name_if(char *name_ID, int *err, int len)
void hecmw_restart_add_int_if(int *data, int *n_data, int *err)
void hecmw_restart_write_if(int *err)
integer(kind=kint) function hecmw_comm_get_comm()
void hecmw_restart_add_real_if(double *data, int *n_data, int *err)
void hecmw_restart_open_if(int *err)