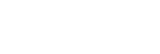 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
64 if (nval > 0 && set->
sorted) {
111 if (index < set->iter) set->
iter--;
134 if (set->
iter == nval) {
struct hecmw_varray_int * vals
void HECMW_set_int_finalize(struct hecmw_set_int *set)
int HECMW_set_int_is_empty(const struct hecmw_set_int *set)
int HECMW_varray_int_search(struct hecmw_varray_int *varray, int value, size_t *index)
void HECMW_set_int_iter_init(struct hecmw_set_int *set)
int HECMW_set_int_del(struct hecmw_set_int *set, int value)
#define HECMW_malloc(size)
int HECMW_varray_int_delete(struct hecmw_varray_int *varray, size_t index)
void HECMW_varray_int_sort(struct hecmw_varray_int *varray)
int HECMW_set_int_add(struct hecmw_set_int *set, int value)
size_t HECMW_set_int_check_dup(struct hecmw_set_int *set)
int HECMW_varray_int_get(const struct hecmw_varray_int *varray, size_t index)
size_t HECMW_set_int_nval(struct hecmw_set_int *set)
int HECMW_set_int_init(struct hecmw_set_int *set)
void HECMW_varray_int_finalize(struct hecmw_varray_int *varray)
int HECMW_varray_int_init(struct hecmw_varray_int *varray)
int HECMW_set_int_iter_next(struct hecmw_set_int *set, int *value)
int HECMW_varray_int_append(struct hecmw_varray_int *varray, int value)
size_t HECMW_varray_int_nval(const struct hecmw_varray_int *varray)
#define HECMW_assert(cond)
size_t HECMW_varray_int_uniq(struct hecmw_varray_int *varray)