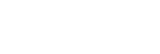 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
25 static int set_n(
void *src) {
26 couple_value->
n = *((
int *)src);
30 static int set_item_type(
void *src) {
35 static int set_n_dof(
void *src) {
36 couple_value->
n_dof = *((
int *)src);
40 static int set_item(
void *src) {
43 if (couple_value->
n <= 0)
return 0;
46 size =
sizeof(*couple_value->
item) * couple_value->
n;
48 size =
sizeof(*couple_value->
item) * couple_value->
n;
50 size =
sizeof(*couple_value->
item) * couple_value->
n * 2;
59 memcpy(couple_value->
item, src, size);
63 static int set_value(
void *src) {
66 if (couple_value->
n <= 0 || couple_value->
n_dof <= 0)
return 0;
68 size =
sizeof(*couple_value->
value) * couple_value->
n * couple_value->
n_dof;
74 memcpy(couple_value->
value, src, size);
85 static struct func_table {
91 {
"hecmw_couple_value",
"n", set_n},
92 {
"hecmw_couple_value",
"item_type", set_item_type},
93 {
"hecmw_couple_value",
"n_dof", set_n_dof},
94 {
"hecmw_couple_value",
"item", set_item},
95 {
"hecmw_couple_value",
"value", set_value},
98 static const int NFUNC =
sizeof(functions) /
sizeof(functions[0]);
100 static SetFunc get_set_func(
char *struct_name,
char *var_name) {
103 for (i = 0; i < NFUNC; i++) {
104 if (strcmp(functions[i].struct_name, struct_name) == 0 &&
105 strcmp(functions[i].var_name, var_name) == 0) {
106 return functions[i].set_func;
116 couple_value = _couple_value;
128 void *src,
int *err,
int slen,
int vlen) {
135 if (couple_value ==
NULL) {
138 "hecmw_cpl_copy_f2c_set_if(): 'couple_value' has not initialized yet");
141 if (struct_name ==
NULL) {
143 "hecmw_cpl_copy_f2c_set_if(): 'struct_name' is NULL");
146 if (var_name ==
NULL) {
148 "hecmw_cpl_copy_f2c_set_if(): 'var_name' is NULL");
156 if ((func = get_set_func(sname, vname)) ==
NULL) {
158 "hecmw_cpl_copy_f2c_set_if(): SetFunc not found");
162 if ((*func)(src))
return;
168 void *src,
int *err,
int slen,
174 void *src,
int *err,
int slen,
180 void *src,
int *err,
int slen,
int vlen) {
void HECMW_CPL_COPY_F2C_SET_IF(char *struct_name, char *var_name, void *src, int *err, int slen, int vlen)
int HECMW_couple_copy_f2c_finalize(void)
#define HECMWCPL_E_INVALID_NULL_PTR
#define HECMW_malloc(size)
#define HECMW_COUPLE_ELEMENT_GROUP
void hecmw_cpl_copy_f2c_set_if__(char *struct_name, char *var_name, void *src, int *err, int slen, int vlen)
#define HECMW_COUPLE_NODE_GROUP
int HECMW_couple_copy_f2c_init(struct hecmw_couple_value *_couple_value)
#define HECMW_COUPLE_SURFACE_GROUP
void hecmw_cpl_copy_f2c_set_if_(char *struct_name, char *var_name, void *src, int *err, int slen, int vlen)
char * HECMW_strcpy_f2c_r(const char *fstr, int flen, char *buf, int bufsize)
void hecmw_cpl_copy_f2c_set_if(char *struct_name, char *var_name, void *src, int *err, int slen, int vlen)
int HECMW_set_error(int errorno, const char *fmt,...)
#define HECMWCPL_E_INVALID_ARG