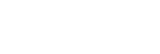 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
35 static int find_edge(
const struct hecmw_graph *graph,
46 static int add_edge_one_way(
struct hecmw_graph *graph,
74 int *edge_index,
int *edge_item) {
117 if (add_edge_one_way(graph, vert1, vert2) ==
HECMW_SUCCESS &&
127 int idx_start, idx_end;
130 fprintf(fp,
"num_edge = %d\n", graph->
m_num_edge);
133 fprintf(fp,
"%d: ", i);
135 idx_start = edge_index[i];
136 idx_end = edge_index[i + 1];
137 for (j = idx_start; j < idx_end; j++) {
138 fprintf(fp,
" %d", edge_item[j]);
162 const int *parttab) {
181 for (i = 0; i < num_part; i++) {
188 start = ref_edge_index[i];
189 end = ref_edge_index[i + 1];
190 for (j = start; j < end; j++) {
191 jj = ref_edge_item[j];
192 j_part = parttab[jj];
193 if (i_part == j_part)
continue;
206 for (i = 0; i < num_part; i++) {
210 edge_index[i + 1] = edge_index[i] + n_edge;
215 for (i = 0; i < num_part; i++) {
216 start = edge_index[i];
218 for (j = 0; j < n_edge; j++) {
223 for (i = 0; i < num_part; i++) {
231 for (i = 0; i < num_part; i++) {
251 int find_edge(
const struct hecmw_graph *graph,
int vert1,
int vert2,
int *idx) {
254 int idx_start, idx_end;
257 idx_start = edge_index[vert1];
258 idx_end = edge_index[vert1 + 1];
259 for (i = idx_start; i < idx_end; i++) {
260 if (edge_item[i] == vert2) {
268 int add_edge_one_way(
struct hecmw_graph *graph,
int vert1,
int vert2) {
276 if (find_edge(graph, vert1, vert2, &idx)) {
int * HECMW_varray_int_get_v(struct hecmw_varray_int *varray)
int HECMW_graph_addEdge(struct hecmw_graph *graph, int vert1, int vert2)
#define HECMW_malloc(size)
void HECMW_varray_int_sort(struct hecmw_varray_int *varray)
int HECMW_varray_int_resize(struct hecmw_varray_int *varray, size_t len)
int HECMW_varray_int_get(const struct hecmw_varray_int *varray, size_t index)
int HECMW_graph_degeneGraph(struct hecmw_graph *graph, const struct hecmw_graph *refgraph, int num_part, const int *parttab)
int HECMW_graph_getNumVertex(const struct hecmw_graph *graph)
int HECMW_varray_int_assign(struct hecmw_varray_int *varray, size_t begin, size_t end, int val)
void HECMW_graph_finalize(struct hecmw_graph *graph)
struct hecmw_varray_int * m_edge_index
struct hecmw_varray_int * m_edge_item
void HECMW_varray_int_finalize(struct hecmw_varray_int *varray)
int HECMW_varray_int_init(struct hecmw_varray_int *varray)
void HECMW_graph_setNumVertex(struct hecmw_graph *graph, int num_vertex)
const int * HECMW_graph_getEdgeItem(const struct hecmw_graph *graph)
int HECMW_graph_getNumEdge(const struct hecmw_graph *graph)
int HECMW_graph_init_with_arrays(struct hecmw_graph *graph, int num_vertex, int *edge_index, int *edge_item)
int HECMW_graph_init(struct hecmw_graph *graph)
void HECMW_graph_print(const struct hecmw_graph *graph, FILE *fp)
int HECMW_varray_int_append(struct hecmw_varray_int *varray, int value)
int HECMW_set_error(int errorno, const char *fmt,...)
size_t HECMW_varray_int_nval(const struct hecmw_varray_int *varray)
const int * HECMW_graph_getEdgeIndex(const struct hecmw_graph *graph)
#define HECMW_assert(cond)
size_t HECMW_varray_int_uniq(struct hecmw_varray_int *varray)
int HECMW_varray_int_insert(struct hecmw_varray_int *varray, size_t index, int val)
const int * HECMW_varray_int_get_cv(const struct hecmw_varray_int *varray)