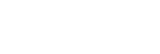 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
71 static int map_resize(
struct hecmw_map_int *map,
size_t new_max_val) {
83 if (new_max_val == 0) {
102 map->
pairs = new_pairs;
119 return map_resize(map, new_max_val);
147 static int pair_cmp(
const void *v1,
const void *v2) {
153 if (p1->
key < p2->
key)
return -1;
154 if (p1->
key > p2->
key)
return 1;
159 size_t i, n_dup = 0, n = 1;
173 for (i = 1; i < map->
n_val; i++) {
192 map_resize(map, map->
n_val);
197 static int map_search(
const struct hecmw_map_int *map,
int key,
size_t *index) {
198 size_t left, right, center;
206 right = map->
n_val - 1;
208 while (left <= right) {
209 center = (left + right) / 2;
332 for (i = 0; i < map->
n_val; i++) {
349 for (i = 0; i < map->
n_val; i++) {
351 if (n_del > 0) map->
vals[i - n_del] = map->
vals[i];
int HECMW_map_int_add(struct hecmw_map_int *map, int key, void *value)
int HECMW_map_int_iter_next(struct hecmw_map_int *map, int *key, void **value)
int HECMW_bit_array_get(struct hecmw_bit_array *ba, size_t index)
#define HECMW_realloc(ptr, size)
void HECMW_map_int_iter_init(struct hecmw_map_int *map)
void HECMW_bit_array_unset(struct hecmw_bit_array *ba, size_t index)
#define HECMW_malloc(size)
int HECMW_map_int_iter_next_unmarked(struct hecmw_map_int *map, int *key, void **value)
size_t HECMW_map_int_nval(const struct hecmw_map_int *map)
int HECMW_map_int_key2local(const struct hecmw_map_int *map, int key, size_t *local)
struct hecmw_map_int_value * vals
int HECMW_map_int_init(struct hecmw_map_int *map, void(*free_fnc)(void *))
void HECMW_bit_array_set_all(struct hecmw_bit_array *ba)
struct hecmw_bit_array * mark
int HECMW_map_int_mark_init(struct hecmw_map_int *map)
int HECMW_bit_array_init(struct hecmw_bit_array *ba, size_t len)
void * HECMW_map_int_get(const struct hecmw_map_int *map, int key)
int HECMW_map_int_mark(struct hecmw_map_int *map, int key)
void HECMW_bit_array_finalize(struct hecmw_bit_array *ba)
size_t HECMW_map_int_check_dup(struct hecmw_map_int *map)
int HECMW_map_int_del_unmarked(struct hecmw_map_int *map)
void HECMW_bit_array_set(struct hecmw_bit_array *ba, size_t index)
void HECMW_map_int_finalize(struct hecmw_map_int *map)
#define HECMW_assert(cond)
struct hecmw_map_int_pair * pairs