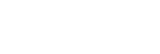 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
6 #ifndef HECMW_VIS_RESAMPLING_H_INCLUDED
7 #define HECMW_VIS_RESAMPLING_H_INCLUDED
23 #define HEX_NODE_INDEX 255
24 #define HEX_FACE_INDEX 63
26 #define MAX_LINE_LEN 256
struct cube_head_struct Cube_head
struct extent_data Extent_data
struct cube_pointer_struct * cube_link
struct in_surface In_surface
struct cont_data Cont_data
struct surfacep_info Surfacep_info
struct surfacep_info * next_patch
struct voxel_info Voxel_info
struct cube_pointer_struct Cube_point
struct voxel_data Voxel_data
Surfacep_info * next_patch
struct cube_pointer_struct * next_point
struct head_surface_info Head_surfacep_info