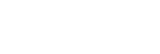 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
21 static int is_valid_label(
char *label) {
22 #define ALLOW_CHAR_FIRST "_"
23 #define ALLOW_CHAR "_-+"
27 if (label ==
NULL)
return 0;
97 static int setup_MPC(
int n_elem_type,
int *elem_type_index,
int *elem_type_item,
99 int itype, ic_type, is, ie, icel;
106 for (itype = 0; itype < n_elem_type; itype++) {
107 ic_type = elem_type_item[itype];
123 if (elemID_wo_MPC ==
NULL) {
129 for (itype = 0; itype < n_elem_type; itype++) {
130 ic_type = elem_type_item[itype];
136 is = elem_type_index[itype];
137 ie = elem_type_index[itype + 1];
138 for (icel = is; icel < ie; icel++) {
148 elemID_wo_MPC[nelem_wo_MPC] = elemID[icel];
162 int n_elem_type,
int *elem_type_index,
int *elem_type_item,
163 int i_step,
char *header,
char *comment) {
175 if (header ==
NULL) {
183 while (len <
sizeof(
ResIO.
head) - 1 && *p && *p !=
'\n') {
189 if (comment ==
NULL) {
203 rtc = setup_MPC(n_elem_type, elem_type_index, elem_type_item, elemID);
213 static int add_to_global_list(
struct result_list *result) {
228 static int add_to_node_list(
struct result_list *result) {
243 static int add_to_elem_list(
struct result_list *result) {
264 if (result ==
NULL) {
270 if (new_label ==
NULL) {
275 result->
label = new_label;
298 if (!is_valid_label(
label)) {
310 size =
sizeof(double) * n *
n_dof;
320 for (idof = 0; idof <
n_dof; idof++) {
329 if (result ==
NULL) {
334 if (add_to_node_list(result))
goto error;
336 if (add_to_elem_list(result))
goto error;
338 if (add_to_global_list(result))
goto error;
void HECMW_result_io_finalize()
@ HECMW_RESULT_DTYPE_NODE
struct result_list * node_list
#define HECMW_malloc(size)
int HECMW_log(int loglv, const char *fmt,...)
int HECMW_result_io_count_ng_comp(void)
#define HECMW_calloc(nmemb, size)
struct result_list * next
char head[HECMW_HEADER_LEN+1]
int HECMW_result_io_add(int dtype, int n_dof, char *label, double *ptr)
int HECMW_is_etype_link(int etype)
struct result_list * elem_list
int HECMW_result_io_count_ne_comp(void)
struct result_list * global_list
int HECMW_is_etype_patch(int etype)
struct hecmwST_result_io_data ResIO
int HECMW_result_io_init(int n_node, int n_elem, int *nodeID, int *elemID, int n_elem_type, int *elem_type_index, int *elem_type_item, int i_step, char *header, char *comment)
@ HECMW_RESULT_DTYPE_ELEM
char comment_line[HECMW_MSG_LEN+1]
int HECMW_is_etype_smoothing(int etype)
int HECMW_set_error(int errorno, const char *fmt,...)
int HECMW_result_io_count_nn_comp(void)