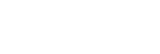 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
20 #define AUTHOR "The University of Tokyo, RSS21 Project"
23 static bool fg_hecmw_ctrl =
false;
24 static bool fg_res_name =
false;
25 static bool fg_vis_name =
false;
26 static char date_time[256];
27 static char neu_name[256];
28 static char mesh_name[256];
29 static char ctrl_name[256];
30 static char hecmw_ctrl_name[256] =
"hecmw_ctrl.dat";
31 static char res_name[256];
32 static char vis_name[256];
33 static bool fg_direct =
false;
40 static void remove_cr(
char *s) {
47 if (s[n] ==
'\r' || s[n] ==
'\n') s[n] = 0;
53 printf(
"NEU to FrontSTR Data Converter, Ver. %s \n",
VER);
54 printf(
"Copyright (C) 2006 %s, All right reserved.\n",
AUTHOR);
62 " neu2fstr ([options]) [sSeEhH] [neu] [mesh] [ctrl]\n"
64 " -h : show help (this message)\n"
65 " -c : create HEC-MW control file (hecmw_ctrl.dat)\n"
66 " -r [name] : write result file name in hecmw_ctrl.dat "
67 "(default:[mesh].res)\n"
68 " -v [name] : write visual file name in hecmw_ctrl.dat "
69 "(default:[mesh].vis)\n"
70 " -d : set direct solver in FrontSTR control file\n"
71 " [sSeEhH] : command character s,S,e,E,h or H (specify only one "
73 " s,S : generate for static analysis\n"
74 " e,E : generate for eigen analysis\n"
75 " CAUTION) Edit !EIGEN section in generated [ctrl]\n"
76 " h,H : generate for heat transfer analysis\n"
77 " [neu] : neutral file name\n"
78 " [mesh] : mesh file name\n"
79 " [ctrl] : FrontSTR control file name\n");
87 #define CMP(x) (strcmp(argv[i], (x)) == 0)
89 for (
int i = 1; i < argc; i++) {
93 }
else if (
CMP(
"-c")) {
96 }
else if (
CMP(
"-d")) {
99 }
else if (
CMP(
"-v")) {
104 fprintf(stderr,
"##Error: Visual output file name is required\n");
108 strcpy(vis_name, argv[i]);
110 }
else if (
CMP(
"-r")) {
115 fprintf(stderr,
"##Error: Result file name is required\n");
119 strcpy(res_name, argv[i]);
124 if (
CMP(
"s") ||
CMP(
"S")) {
127 }
else if (
CMP(
"e") ||
CMP(
"E")) {
130 }
else if (
CMP(
"h") ||
CMP(
"H")) {
134 fprintf(stderr,
"##Error: No such solution type %s\n", argv[i]);
139 strcpy(neu_name, argv[i]);
143 strcpy(mesh_name, argv[i]);
147 strcpy(ctrl_name, argv[i]);
151 fprintf(stderr,
"##Error: Too many arguments\n");
164 "##Error: one character, neu, mesh and ctrl file must be "
169 fprintf(stderr,
"##Error: neu, mesh and ctrl file must be specified\n");
173 fprintf(stderr,
"##Error: mesh and ctrl file must be specified\n");
177 fprintf(stderr,
"##Error: ctrl file must be specified\n");
182 strcpy(res_name, mesh_name);
183 strcat(res_name,
".res");
187 strcpy(vis_name, mesh_name);
188 strcat(vis_name,
".vis");
199 strcat(s,
"###########################################################\n");
202 sprintf(ss,
" HEC-MW Mesh File Generated by neu2fstr ver.%s\n",
VER);
205 sprintf(ss,
" FrontSTR Control File Generated by neu2fstr ver.%s\n",
VER);
209 sprintf(ss,
" Date&Time: %s\n", date_time);
211 sprintf(ss,
" Original : %s\n", neu_name);
215 sprintf(ss,
" Control : %s\n", ctrl_name);
218 sprintf(ss,
" Mesh : %s\n", mesh_name);
222 strcat(s,
"###########################################################\n");
228 FILE *fp = fopen(hecmw_ctrl_name,
"w");
231 fprintf(stderr,
"##Error: Cannot create hecmw_ctrl.dat file\n");
235 fprintf(fp,
"###########################################################\n");
236 fprintf(fp,
"# HEC-MW Control File Generated by neu2fstr ver.%s\n",
VER);
237 fprintf(fp,
"# Date&Time: %s\n", date_time);
238 fprintf(fp,
"# Original : %s\n", neu_name);
239 fprintf(fp,
"###########################################################\n");
240 fprintf(fp,
"!MESH, NAME=fstrMSH,TYPE=HECMW-ENTIRE\n");
241 fprintf(fp,
" %s\n", mesh_name);
242 fprintf(fp,
"!CONTROL,NAME=fstrCNT\n");
243 fprintf(fp,
" %s\n", ctrl_name);
244 fprintf(fp,
"!RESULT,NAME=fstrRES,IO=OUT\n");
245 fprintf(fp,
" %s\n", res_name);
246 fprintf(fp,
"!RESULT,NAME=vis_out,IO=OUT\n");
247 fprintf(fp,
" %s\n", vis_name);
274 fstrdata.
DB.push_back(sol);
283 strcpy(solver->
method,
"DIRECT");
286 strcpy(solver->
method,
"CG");
289 fstrdata.
DB.push_back(solver);
296 int main(
int argc,
char **argv) {
299 strcpy(date_time, ctime(&t));
300 remove_cr(date_time);
309 cout <<
"loading neu file ... " << endl;
312 nfdata.
Load(neu_name);
315 fprintf(stderr,
"NEU loading error : %s\n", e.
Msg());
320 cout <<
"converting to HEC-MW mesh ... " << endl;
322 cout <<
"converting to FrontSTR control data " << endl;
330 fprintf(stderr,
"Converting error : %s\n", e.
Msg());
335 cout <<
"saving HEC-MW mesh file..." << endl;
338 if (!fstrdata.
SaveMesh(mesh_name, comment)) {
339 fprintf(stderr,
"Cannot save mesh data\n");
343 cout <<
"saving FrontSTR control file..." << endl;
346 if (!fstrdata.
SaveCtrl(ctrl_name, comment)) {
347 fprintf(stderr,
"Cannot save control data\n");
352 cout <<
"creating hecmw_ctrl.dat file..." << endl;
357 cout <<
"neu2fstr completed." << endl;
void create_comment(char *s, bool fg_mesh)
virtual bool SaveMesh(const char *file_name, const char *comment="")
virtual bool SaveCtrl(const char *file_name, const char *comment="")
void conv_neu2hec(CNFData &neu, CHECData &hec, int sol)
void set_solution(CFSTRData &fstrdata, int solution)
virtual const char * Msg()
bool conv_neu2fstr_dynamic(CNFData &neu, CHECData &hec)
void conv_neu2fstr_heat(CNFData &neu, CHECData &hec)
void conv_neu2fstr_static(CNFData &neu, CHECData &hec)
std::vector< CHECDataBlock * > DB
bool set_params(int argc, char **argv)
virtual const char * Msg()
virtual void Load(const char *fname)
void set_solver(CFSTRData &fstrdata, bool fg_direct=false)
int main(int argc, char **argv)