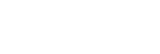 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
20 static int my_comp(
const void *a,
const void *b) {
21 return (*(
int *)a - *(
int *)b);
25 qsort(index_data, n,
sizeof(
int) * 2, my_comp);
void FSTR_SORT_INDEX__(int *index_data, int *n)
void FSTR_SORT_INDEX(int *index_data, int *n)
void FSTR_SORT_INDEX_(int *index_data, int *n)
void fstr_sort_index_(int *index_data, int *n)
void fstr_sort_index(int *index_data, int *n)
void c_fstr_sort_index(int *index_data, int n)
I/O and Utility.
void fstr_sort_index__(int *index_data, int *n)