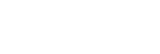 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
34 static int fg_little_endian =
TRUE;
36 #define RES_BIN_HEADER "HECMW_BINARY_RESULT"
45 static void check_endian(
void) {
50 fg_little_endian = (u.
c[0] == 1);
61 if (fg_little_endian) {
64 for (i = 0; i < size; i++) {
65 if (putc(*
c, fp) == EOF)
return FAIL;
74 for (i = 0; i < size; i++) {
75 if (putc(*
c, fp) == EOF)
return FAIL;
93 for (i = 0; i < n; i++, x++) {
111 for (i = 0; i < n; i++, x++) {
127 if (fg_little_endian) {
130 for (i = 0; i < size; i++) {
135 *
c = (
unsigned char)
data;
143 for (i = 0; i < size; i++) {
148 *
c = (
unsigned char)
data;
171 for (i = 0; i < n; i++, x++) {
194 for (i = 0; i < n; i++, x++) {
205 static int get_fmt_type_size(
char **fmt_p,
char *type,
int *size) {
217 while (*p &&
'0' <= *p && *p <=
'9') {
228 sscanf(
s,
"%d", size);
252 n = strlen((
char *)fmt);
256 while (get_fmt_type_size(&fmt_p, &type, &size)) {
261 i_ptr = va_arg(va,
int *);
266 i_data = va_arg(va,
int);
276 d_ptr = va_arg(va,
double *);
281 d_data = va_arg(va,
double);
291 s_ptr = va_arg(va,
char *);
293 if (fwrite(s_ptr,
sizeof(
char), size, fp) != size)
goto ERROR_EXIT;
296 s_ptr = va_arg(va,
char *);
299 if (fwrite(s_ptr,
sizeof(
char), 1, fp) != 1)
goto ERROR_EXIT;
304 if (fwrite(s_ptr,
sizeof(
char), 1, fp) != 1)
goto ERROR_EXIT;
310 fprintf(stderr,
"Illeagal type : %c (0x%x)\n", type, type);
333 n = strlen((
char *)fmt);
337 while (get_fmt_type_size(&fmt_p, &type, &size)) {
341 if (size == 0) size = 1;
343 i_ptr = va_arg(va,
int *);
351 if (size == 0) size = 1;
353 d_ptr = va_arg(va,
double *);
362 s_ptr = va_arg(va,
char *);
364 if (fread(s_ptr,
sizeof(
char), size, fp) != size)
goto ERROR_EXIT;
367 s_ptr = va_arg(va,
char *);
370 if (fread(&
c,
sizeof(
char), 1, fp) != 1)
break;
int hecmw_read_bin_value(unsigned char *x, int size, FILE *fp)
void hecmw_set_endian_info(void)
int hecmw_write_bin_int_arr(int *x, int n, FILE *fp)
int hecmw_read_bin_int(int *x, FILE *fp)
int hecmw_read_bin(FILE *fp, const char *fmt,...)
int hecmw_write_bin_value(unsigned char *x, int size, FILE *fp)
int hecmw_write_bin_double(double x, FILE *fp)
int hecmw_read_bin_double(double *x, FILE *fp)
int hecmw_write_bin(FILE *fp, const char *fmt,...)
int hecmw_read_bin_int_arr(int *x, int n, FILE *fp)
int hecmw_read_bin_double_arr(double *x, int n, FILE *fp)
int hecmw_write_bin_double_arr(double *x, int n, FILE *fp)
int hecmw_write_bin_int(int x, FILE *fp)