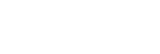 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
6 #define fstr_ctrl_util_MAIN
17 #define STR_SIZE buffsize
18 #define FILE_NAME_SIZE 512
24 static void set_param_err_msg(
fstr_ctrl_data *ctrl,
const char *param_name,
26 static void set_data_err_msg(
fstr_ctrl_data *ctrl,
int line,
int data_no,
28 static void set_record_data_line_err_msg(
fstr_ctrl_data *ctrl,
int r);
29 static char *gettoken(
const char *line);
30 static void strcpy_f2c(
char *dest,
const char *src,
int len);
31 static void strcpy_c2f(
char *dest,
int len,
const char *src);
32 static char *remove_header_space(
char *token);
33 static int Strncmpi(
const char *s1,
const char *s2,
int len);
34 static int Strcmpi(
const char *s1,
const char *s2);
36 static void remove_cr(
char *s);
37 static void format_conv(
const char *format,
char *fmt,
int *array_size);
63 for (i = 0; i < ctrl->
rec_n; i++) {
95 static int is_comment_or_blank_line(
char *buff) {
98 if (buff[0] ==
'\n' || buff[0] == 0 || buff[0] ==
'#' ||
99 (buff[0] ==
'!' && buff[1] ==
'!')) {
104 if (*p !=
' ' && *p !=
'\n') {
116 static int count_line_and_header_number(
const char *fname,
int *line_n,
121 fp = fopen(fname,
"r");
137 if (is_comment_or_blank_line(buff)) {
141 if (buff[0] ==
'!') {
156 static int set_fstr_ctrl_data(
const char *fname,
fstr_ctrl_data *ctrl) {
162 fp = fopen(fname,
"r");
172 while (fgets(buff,
buffsize - 1, fp)) {
175 if (is_comment_or_blank_line(buff)) {
179 if (buff[0] ==
'!') {
185 if (header_count >= 0) {
194 printf(
"Not enough memory\n");
200 strcpy(ctrl->
rec[rec_count].
line, buff);
210 static int create_fstr_ctrl_data(
const char *fname,
fstr_ctrl_data *ctrl) {
212 int line_n, header_n;
213 fstr_ctrl_data_init(ctrl);
215 if (count_line_and_header_number(fname, &line_n, &header_n)) {
219 ctrl->
rec_n = line_n;
222 for (i = 0; i < line_n; i++) {
231 for (i = 0; i < header_n; i++) {
236 if (set_fstr_ctrl_data(fname, ctrl)) {
237 fstr_ctrl_data_finalize(ctrl);
268 err = create_fstr_ctrl_data(filename, ctrl);
285 for (i = 0; i < ctrl->
rec_n; i++) {
289 line_size = strlen(ctrl->
rec[i].
line) + 1;
321 if (create_fstr_ctrl_data(filename, ctrl)) {
349 if (rec_no < 0 || rec_no >= ctrl->
rec_n) {
353 strcpy(buff, ctrl->
rec[rec_no].
line);
362 static char *h_name =
NULL;
370 if (header_name ==
NULL || header_name[0] == 0) {
375 h_name = (
char *)header_name;
378 if (h_name ==
NULL) {
382 header_name_len = strlen(h_name);
384 for (i = start_index; i < ctrl->
header_n; i++) {
386 char *header = ctrl->
rec[hp].
line;
388 if (Strncmpi(header, h_name, header_name_len) == 0) {
430 header_p = header_name;
432 while (*line_p && (*line_p !=
',') && (*line_p !=
' ') && (*line_p !=
'\n') &&
434 *header_p = (char)
toupper(*line_p);
476 static int param_value_check(
const char *value_list,
char *val,
int *index) {
481 if (value_list ==
NULL || value_list[0] == 0 || value_list[0] ==
'#') {
491 n = strlen(value_list);
493 for (i = 0; i < n; i++) {
494 if (value_list[i] !=
' ') {
508 strcpy(vlist, value_list);
510 token = strtok(vlist,
" ,");
513 if (Strcmpi(token, val) == 0) {
521 token = strtok(
NULL,
", ");
528 static int param_value_convert(
int type,
char *token,
void *val) {
537 switch ((
char)type) {
562 r = sscanf(token, fmt, val);
571 static int param_length_count(
const char *param) {
572 const char *p = param;
574 while (*p !=
'\0' && *p !=
'=' && *p !=
' ') {
582 const char *value_list,
char type,
void *val) {
599 strcpy(header, ctrl->
rec[h_pos].
line);
610 param_name_len = strlen(param_name);
611 strtok(header,
",\n");
612 token = strtok(
NULL,
",\n");
615 param_pos = remove_header_space(token);
616 param_len = param_length_count(param_pos);
618 if (param_len == param_name_len && Strncmpi(param_pos, param_name, param_name_len) == 0) {
619 if (type ==
'E' || type ==
'e') {
624 eq_pos = strstr(param_pos,
"=");
627 val_pos = eq_pos + 1;
628 val_pos = remove_header_space(val_pos);
640 if (param_value_check(value_list, val_pos, &index)) {
644 if (type ==
'P' || type ==
'p') {
645 *((
int *)val) = index;
649 r = param_value_convert(type, val_pos, val);
658 token = strtok(
NULL,
",\n");
662 if (type ==
'E' || type ==
'e') {
672 static int rcode_of_get_param = 0;
675 const char *value_list,
int necessity,
char type,
679 rcode_of_get_param = rcode;
687 set_param_err_msg(ctrl, param_name,
" is required");
697 set_param_err_msg(ctrl, param_name,
": value is required");
706 set_param_err_msg(ctrl, param_name,
": type conversion fail");
710 sprintf(s,
": type range fail(%s)", value_list);
711 set_param_err_msg(ctrl, param_name, s);
751 if (data_line_n <= 0 || data_line_n <
line_no) {
757 strcpy(data_line, ctrl->
rec[data_pos].
line);
778 token = strtok(data_line, delim);
782 token = strtok(
NULL, delim);
806 va_start(va, format);
813 const char *format, ...) {
816 va_start(va, format);
821 set_record_data_line_err_msg(ctrl, r);
829 const char *format, va_list va) {
839 char fmt_integer[] =
"%d";
840 char fmt_double[] =
"%lf";
841 char fmt_string[] =
"%s";
842 char fmt_char[] =
"%c";
844 int array_size[
buffsize] = {
'\0' };
846 format_conv(format, format_c, array_size);
853 while (format_c[i] != 0) {
854 if (isupper(format_c[i])) {
864 len = strlen(format_c);
867 if (data_line[0] ==
'!') {
868 for (; counter < len; counter++) {
869 if (isupper(format_c[counter])) {
877 token = gettoken(data_line);
879 while (token && counter < len) {
881 necessary = isupper(format_c[counter]);
882 type =
toupper(format_c[counter]) & 0xff;
884 switch ((
char)type) {
905 val_p = va_arg(va,
void *);
908 err = sscanf(token, fmt, val_p);
910 if (err != 1 && necessary) {
914 if ((
char)type ==
'S') {
915 strcpy(buff, (
char *)val_p);
916 strcpy_c2f((
char *)val_p, array_size[counter], buff);
919 }
else if (necessary) {
923 token = gettoken(
NULL);
927 for (; counter < len; counter++) {
928 if (isupper(format[counter])) {
938 #define MAX_DATA_ARRAY_NUMBER 256
956 format_conv(format, fmt, array_size);
965 param[i] = va_arg(va,
void *);
968 switch ((
char)type) {
970 param_size[i] =
sizeof(int);
974 param_size[i] =
sizeof(double);
978 param_size[i] =
sizeof(char) * array_size[i];
982 param_size[i] =
sizeof(char);
995 for (i = 1; i <= line_n; i++) {
997 param[3], param[4], param[5], param[6], param[7],
998 param[8], param[9], param[10], param[11],
999 param[12], param[13], param[14], param[15],
1000 param[16], param[17], param[18], param[19]);
1007 for (j = 0; j < column_n; j++) {
1009 strcpy(buff, (
char *)param[j]);
1010 strcpy_c2f((
char *)param[j], array_size[j], buff);
1013 param[j] += param_size[j];
1018 #undef MAX_DATA_ARRAY_NUMBER
1024 va_start(va, format);
1034 va_start(va, format);
1039 set_record_data_line_err_msg(ctrl, r);
1051 fstr_ctrl_data_finalize(ctrl);
1059 printf(
"header pos: ");
1061 for (i = 0; i < ctrl->
header_n; i++) {
1067 for (i = 0; i < ctrl->
rec_n; i++) {
1077 strcpy_c2f(f_buff, *len,
err_msg);
1081 strcpy_c2f(f_buff, *len,
err_msg);
1085 strcpy_c2f(f_buff, *len,
err_msg);
1089 strcpy_c2f(f_buff, *len,
err_msg);
1093 strcpy_c2f(f_buff, *len,
err_msg);
1097 strcpy_c2f(f_buff, *len,
err_msg);
1186 strcpy_c2f(buff, *buff_size, c_buff);
1214 strcpy_f2c(name, header_name,
STR_SIZE);
1279 strcpy_c2f(header_name, *buff_size, c_buff);
1358 const char *value_list,
char *type,
void *val) {
1362 memset(p_name,
'\0',
sizeof(p_name));
1363 memset(v_list,
'\0',
sizeof(v_list));
1364 strcpy_f2c(p_name, param_name,
STR_SIZE);
1365 strcpy_f2c(v_list, value_list,
STR_SIZE);
1368 if (rcode == 0 && (*type ==
'S' || *type ==
's')) {
1370 strcpy(buff, (
char *)val);
1378 const char *value_list,
char *type,
void *val) {
1383 const char *value_list,
char *type,
void *val) {
1388 const char *value_list,
char *type,
void *val) {
1393 const char *value_list,
char *type,
void *val) {
1398 const char *value_list,
char *type,
void *val) {
1405 const char *value_list,
int *necessity,
char *type,
1410 strcpy_f2c(p_name, param_name,
STR_SIZE);
1411 strcpy_f2c(v_list, value_list,
STR_SIZE);
1416 (*type ==
'S' || *type ==
's')) {
1418 strcpy(buff, (
char *)val);
1426 const char *value_list,
int *necessity,
char *type,
1433 const char *value_list,
int *necessity,
char *type,
1440 const char *value_list,
int *necessity,
char *type,
1447 const char *value_list,
int *necessity,
char *type,
1454 const char *value_list,
int *necessity,
char *type,
1490 strcpy_f2c(delim_c, delim,
STR_SIZE);
1552 const char *format, va_list va) {
1554 strcpy_f2c(fmt_c, format,
STR_SIZE);
1561 va_start(va, format);
1570 va_start(va, format);
1579 va_start(va, format);
1588 va_start(va, format);
1597 va_start(va, format);
1606 va_start(va, format);
1614 const char *format, va_list va) {
1617 strcpy_f2c(fmt_c, format,
STR_SIZE);
1621 set_record_data_line_err_msg(ctrl, r);
1631 va_start(va, format);
1640 va_start(va, format);
1649 va_start(va, format);
1658 va_start(va, format);
1667 va_start(va, format);
1676 va_start(va, format);
1688 strcpy_f2c(fmt_c, format,
STR_SIZE);
1694 set_record_data_line_err_msg(ctrl, r);
1704 va_start(va, format);
1713 va_start(va, format);
1722 va_start(va, format);
1731 va_start(va, format);
1740 va_start(va, format);
1749 va_start(va, format);
1790 static void set_param_err_msg(
fstr_ctrl_data *ctrl,
const char *param_name,
1792 sprintf(
err_msg,
"fstr control file error(param): line:%d, %s, %s\n",
1798 static void set_data_err_msg(
fstr_ctrl_data *ctrl,
int line,
int data_no,
1801 sprintf(
err_msg,
"fstr control file error(data): line:%d, column:%d : %s\n",
1802 line, data_no, msg);
1805 static void set_record_data_line_err_msg(
fstr_ctrl_data *ctrl,
int r) {
1812 strcpy(msg,
"no error");
1816 strcpy(msg,
"data type converting error");
1820 strcpy(msg,
"data range error");
1824 strcpy(msg,
"data must exist");
1828 strcpy(msg,
"data line does not exist");
1832 sprintf(msg,
"data line unknown error (r:%d)", r);
1835 set_data_err_msg(ctrl,
line_no, pos, msg);
1840 static char *gettoken(
const char *line) {
1853 if (*p == 0 || *p ==
'\n' || *p ==
'\r') {
1859 if (*p == 0 || *p ==
'\n' || *p ==
'\r' || *p ==
',') {
1877 while (*t ==
' ' && t != h) {
1904 static void strcpy_f2c(
char *dest,
const char *src,
int len) {
1908 for (i = 0; i < len - 1; i++) {
1909 if (src[i] !=
' ') {
1924 static void strcpy_c2f(
char *dest,
int len,
const char *src) {
1928 for (i = 0; i < len; i++) {
1929 if (fg == 0 && src[i] == 0) {
1944 static char *remove_header_space(
char *token) {
1960 static int Strncmpi(
const char *s1,
const char *s2,
int len) {
1967 while (*p1 && *p2 && i < len) {
1980 static int Strcmpi(
const char *s1,
const char *s2) {
1985 while (*p1 && *p2) {
2005 void Strupr(
char *s) {
2015 static void remove_cr(
char *s) {
2017 if (*s ==
'\n' || *s ==
'\r') {
2028 static void format_conv(
const char *format,
char *fmt,
int *array_size) {
2029 char *p = (
char *)format;
2032 char num_str[
buffsize] = {
'\0' } ;
2037 if (
'0' <= *p && *p <=
'9') {
2039 num_str[num_index] = *p;
2048 num_str[num_index] = 0;
2049 sscanf(num_str,
"%d", &n);
2050 array_size[i - 1] = n;
2070 num_str[num_index] = 0;
2071 sscanf(num_str,
"%d", &n);
2072 array_size[i - 1] = n;
int FSTR_CTRL_SEEK_HEADER(int *ctrl, const char *header_name)
int fstr_ctrl_get_data_ex__(int *ctrl, int *line_no, const char *format,...)
int c_fstr_ctrl_get_current_header_name(fstr_ctrl_data *ctrl, char *header_name)
void fstr_ctrl_get_err_msg_(char *f_buff, int *len)
int FSTR_CTRL_GET_DATA_LINE_N__(int *ctrl)
int FSTR_CTRL_SEEK_NEXT_HEADER_(int *ctrl)
int FSTR_CTRL_GET_DATA_EX__(int *ctrl, int *line_no, const char *format,...)
int FSTR_CTRL_GET_LINE_(int *ctrl, int *rec_no, char *buff, int *buff_size)
int fstr_ctrl_get_rec_number_(int *ctrl)
int FSTR_CTRL_CLOSE__(int *ctrl)
int FSTR_CTRL_GET_C_H_NAME(int *ctrl, char *header_name, int *buff_size)
int fstr_ctrl_get_data_array_ex_v_f(fstr_ctrl_data *ctrl, const char *format, va_list va)
int FSTR_CTRL_SEEK_HEADER_(int *ctrl, const char *header_name)
int FSTR_CTRL_GET_REC_NUMBER_(int *ctrl)
int FSTR_CTRL_GET_C_H_LINE_NO_(int *ctrl)
int fstr_ctrl_get_param__(int *ctrl, const char *param_name, const char *value_list, char *type, void *val)
int FSTR_CTRL_GET_C_H_LINE_NO(int *ctrl)
#define HECMW_malloc(size)
#define MAX_DATA_ARRAY_NUMBER
#define FSTR_CTRL_RCODE_DATA_NOTHING
void c_fstr_ctrl_dump(fstr_ctrl_data *ctrl)
int fstr_ctrl_get_data_v_f(fstr_ctrl_data *ctrl, int line_no, const char *format, va_list va)
int FSTR_CTRL_GET_C_H_POS(int *ctrl)
int FSTR_CTRL_GET_LINE(int *ctrl, int *rec_no, char *buff, int *buff_size)
int fg_fortran_get_data_array_v
HECMW_Comm HECMW_comm_get_comm(void)
int fstr_ctrl_get_data_n_in_line_(int *ctrl, int *line_no, const char *delim)
void fstr_ctrl_get_err_msg(char *f_buff, int *len)
#define FSTR_CTRL_RCODE_PARAM_RANGE_ERROR
int fstr_ctrl_get_line_(int *ctrl, int *rec_no, char *buff, int *buff_size)
int FSTR_CTRL_GET_DATA_ARRAY_EX__(int *ctrl, const char *format,...)
int fstr_ctrl_get_rec_number(int *ctrl)
integer(kind=kint) myrank
PARALLEL EXECUTION.
void c_fstr_ctrl_get_err_msg(char *buff)
int fstr_ctrl_get_data_error_pos_(void)
int fstr_ctrl_get_c_h_line_no(int *ctrl)
int FSTR_CTRL_REWIND_(int *ctrl)
int fstr_ctrl_close(int *ctrl)
int fstr_ctrl_get_c_h_pos(int *ctrl)
int c_fstr_ctrl_get_current_header_line_no(fstr_ctrl_data *ctrl)
int fstr_ctrl_close__(int *ctrl)
fstr_ctrl_data * c_fstr_ctrl_open(const char *filename)
int HECMW_comm_get_rank(void)
int FSTR_CTRL_GET_DATA_LINE_N(int *ctrl)
int FSTR_CTRL_GET_DATA_ERROR_(void)
int c_fstr_ctrl_get_data_array(fstr_ctrl_data *ctrl, const char *format,...)
#define FSTR_CTRL_RCODE_DATA_SUCCESS
int fstr_ctrl_open_(char *filename)
int fstr_ctrl_get_data_error_line__(void)
int FSTR_CTRL_GET_PARAM__(int *ctrl, const char *param_name, const char *value_list, char *type, void *val)
int fstr_ctrl_get_data_error_pos(void)
int FSTR_CTRL_GET_PARAM_EX(int *ctrl, const char *param_name, const char *value_list, int *necessity, char *type, void *val)
int FSTR_CTRL_CLOSE_(int *ctrl)
#define FSTR_CTRL_RCODE_PARAM_NOTHING
int fstr_ctrl_get_data_ex_(int *ctrl, int *line_no, const char *format,...)
int fstr_ctrl_get_param_ex__(int *ctrl, const char *param_name, const char *value_list, int *necessity, char *type, void *val)
int fstr_ctrl_get_data_error_line_(void)
int fstr_ctrl_get_data_line_n__(int *ctrl)
int c_fstr_ctrl_get_data_array_ex(fstr_ctrl_data *ctrl, const char *format,...)
int FSTR_CTRL_REWIND(int *ctrl)
fstr_ctrl_data * ctrl_list[ctrl_list_size]
int fstr_ctrl_get_param(int *ctrl, const char *param_name, const char *value_list, char *type, void *val)
void fstr_ctrl_dump(int *ctrl)
int fstr_ctrl_get_c_h_name(int *ctrl, char *header_name, int *buff_size)
int FSTR_CTRL_GET_DATA_ERROR_LINE(void)
int FSTR_CTRL_GET_DATA_N_IN_LINE(int *ctrl, int *line_no, const char *delim)
int FSTR_CTRL_GET_C_H_NAME_(int *ctrl, char *header_name, int *buff_size)
int FSTR_CTRL_GET_REC_NUMBER(int *ctrl)
int FSTR_CTRL_REWIND__(int *ctrl)
int c_fstr_ctrl_get_data_error_line(void)
int fstr_ctrl_open(char *filename)
int fstr_ctrl_get_data_line_n_(int *ctrl)
int fstr_ctrl_rewind_(int *ctrl)
int c_fstr_ctrl_get_data(fstr_ctrl_data *ctrl, int line_no, const char *format,...)
int fstr_ctrl_get_c_h_pos__(int *ctrl)
int FSTR_CTRL_SEEK_HEADER__(int *ctrl, const char *header_name)
int fstr_ctrl_get_data_array_ex_(int *ctrl, const char *format,...)
int FSTR_CTRL_GET_DATA_ERROR__(void)
int fstr_ctrl_open__(char *filename)
int FSTR_CTRL_GET_DATA_N_IN_LINE_(int *ctrl, int *line_no, const char *delim)
#define FSTR_CTRL_RCODE_PARAM_TYPE_ERROR
int fstr_ctrl_get_data_n_in_line__(int *ctrl, int *line_no, const char *delim)
int FSTR_CTRL_OPEN__(char *filename)
int FSTR_CTRL_GET_DATA_EX_(int *ctrl, int *line_no, const char *format,...)
int c_fstr_ctrl_get_param(fstr_ctrl_data *ctrl, const char *param_name, const char *value_list, char type, void *val)
void FSTR_CTRL_DUMP_(int *ctrl)
int fstr_ctrl_seek_next_header__(int *ctrl)
int fstr_ctrl_seek_next_header(int *ctrl)
int fstr_ctrl_get_c_h_pos_(int *ctrl)
int fstr_ctrl_get_data_line_n(int *ctrl)
int fstr_ctrl_get_param_(int *ctrl, const char *param_name, const char *value_list, char *type, void *val)
int fstr_ctrl_get_rec_number__(int *ctrl)
int fstr_ctrl_get_c_h_line_no_(int *ctrl)
#define FSTR_CTRL_RCODE_DATA_RANGE_ERROR
int c_fstr_ctrl_get_data_ex(fstr_ctrl_data *ctrl, int line_no, const char *format,...)
int fstr_ctrl_tmp_rewind(fstr_ctrl_data *ctrl)
#define FSTR_CTRL_RCODE_PARAM_SUCCESS
#define FSTR_CTRL_RCODE_DATA_LINE_NOTHING
int FSTR_CTRL_GET_C_H_LINE_NO__(int *ctrl)
int FSTR_CTRL_GET_PARAM_(int *ctrl, const char *param_name, const char *value_list, char *type, void *val)
int fstr_ctrl_get_line__(int *ctrl, int *rec_no, char *buff, int *buff_size)
int FSTR_CTRL_GET_DATA_EX(int *ctrl, int *line_no, const char *format,...)
int fstr_ctrl_get_data_error_pos__(void)
int c_fstr_ctrl_get_param_ex(fstr_ctrl_data *ctrl, const char *param_name, const char *value_list, int necessity, char type, void *val)
void FSTR_CTRL_GET_ERR_MSG__(char *f_buff, int *len)
void FSTR_CTRL_DUMP(int *ctrl)
int FSTR_CTRL_CLOSE(int *ctrl)
void fstr_ctrl_dump_(int *ctrl)
int FSTR_CTRL_GET_DATA_N_IN_LINE__(int *ctrl, int *line_no, const char *delim)
int fstr_ctrl_get_data_error_line(void)
#define FSTR_CTRL_RCODE_DATA_ERROR
int fstr_ctrl_get_data_n_in_line(int *ctrl, int *line_no, const char *delim)
int FSTR_CTRL_GET_DATA(int *ctrl, int *line_no, const char *format,...)
int fstr_ctrl_get_param_ex(int *ctrl, const char *param_name, const char *value_list, int *necessity, char *type, void *val)
int c_fstr_ctrl_seek_next_header(fstr_ctrl_data *ctrl)
int FSTR_CTRL_GET_DATA_LINE_N_(int *ctrl)
int fstr_ctrl_seek_header_(int *ctrl, const char *header_name)
int c_fstr_ctrl_get_data_array_v(fstr_ctrl_data *ctrl, const char *format, va_list va)
int c_fstr_ctrl_get_line(fstr_ctrl_data *ctrl, int rec_no, char *buff)
int FSTR_CTRL_SEEK_NEXT_HEADER__(int *ctrl)
int fstr_ctrl_get_data_ex_v_f(fstr_ctrl_data *ctrl, int line_no, const char *format, va_list va)
void FSTR_CTRL_GET_ERR_MSG_(char *f_buff, int *len)
int c_fstr_ctrl_copy_data_line(fstr_ctrl_data *ctrl, int line_no, char *data_line)
int FSTR_CTRL_GET_C_H_POS__(int *ctrl)
int c_fstr_ctrl_seek_header(fstr_ctrl_data *ctrl, const char *header_name)
int fstr_ctrl_get_param_ex_(int *ctrl, const char *param_name, const char *value_list, int *necessity, char *type, void *val)
int fstr_ctrl_seek_header__(int *ctrl, const char *header_name)
int FSTR_CTRL_GET_PARAM_EX_(int *ctrl, const char *param_name, const char *value_list, int *necessity, char *type, void *val)
int FSTR_CTRL_GET_DATA_ERROR_LINE_(void)
int c_fstr_ctrl_get_data_error_pos(void)
void FSTR_CTRL_GET_ERR_MSG(char *f_buff, int *len)
int fstr_ctrl_get_line(int *ctrl, int *rec_no, char *buff, int *buff_size)
int HECMW_Bcast(void *buffer, int count, HECMW_Datatype datatype, int root, HECMW_Comm comm)
int fstr_ctrl_seek_header(int *ctrl, const char *header_name)
int fstr_ctrl_get_data_array_ex(int *ctrl, const char *format,...)
int fstr_ctrl_get_c_h_name__(int *ctrl, char *header_name, int *buff_size)
int FSTR_CTRL_GET_REC_NUMBER__(int *ctrl)
int FSTR_CTRL_GET_PARAM(int *ctrl, const char *param_name, const char *value_list, char *type, void *val)
int fstr_ctrl_get_data_ex(int *ctrl, int *line_no, const char *format,...)
int fstr_ctrl_get_data__(int *ctrl, int *line_no, const char *format,...)
int fstr_ctrl_get_data_array_ex__(int *ctrl, const char *format,...)
int FSTR_CTRL_GET_DATA_ARRAY_EX(int *ctrl, const char *format,...)
void fstr_ctrl_get_err_msg__(char *f_buff, int *len)
int FSTR_CTRL_GET_LINE__(int *ctrl, int *rec_no, char *buff, int *buff_size)
int c_fstr_ctrl_close(fstr_ctrl_data *ctrl)
int c_fstr_ctrl_get_data_n_in_line(fstr_ctrl_data *ctrl, int line_no, const char *delim)
int fstr_ctrl_get_data(int *ctrl, int *line_no, const char *format,...)
int FSTR_CTRL_GET_DATA_(int *ctrl, int *line_no, const char *format,...)
int FSTR_CTRL_GET_PARAM_EX__(int *ctrl, const char *param_name, const char *value_list, int *necessity, char *type, void *val)
int c_fstr_ctrl_get_rec_number(fstr_ctrl_data *ctrl)
int FSTR_CTRL_GET_DATA_ARRAY_EX_(int *ctrl, const char *format,...)
int fstr_ctrl_seek_next_header_(int *ctrl)
int FSTR_CTRL_OPEN(char *filename)
#define FSTR_CTRL_RCODE_DATA_TYPE_ERROR
#define FSTR_CTRL_RCODE_PARAM_VALUE_NOTHING
int fstr_ctrl_get_c_h_name_(int *ctrl, char *header_name, int *buff_size)
int c_fstr_ctrl_get_data_line_n(fstr_ctrl_data *ctrl)
void FSTR_CTRL_DUMP__(int *ctrl)
int c_fstr_ctrl_get_data_v(fstr_ctrl_data *ctrl, int line_no, const char *format, va_list va)
int FSTR_CTRL_SEEK_NEXT_HEADER(int *ctrl)
int fstr_ctrl_get_c_h_line_no__(int *ctrl)
int fstr_ctrl_rewind(int *ctrl)
void fstr_ctrl_dump__(int *ctrl)
int fstr_ctrl_rewind__(int *ctrl)
int FSTR_CTRL_GET_C_H_NAME__(int *ctrl, char *header_name, int *buff_size)
int FSTR_CTRL_GET_DATA__(int *ctrl, int *line_no, const char *format,...)
int FSTR_CTRL_GET_C_H_POS_(int *ctrl)
int fstr_ctrl_close_(int *ctrl)
int FSTR_CTRL_OPEN_(char *filename)
int c_fstr_ctrl_get_current_header_pos(fstr_ctrl_data *ctrl)
int fstr_ctrl_get_data_(int *ctrl, int *line_no, const char *format,...)
int FSTR_CTRL_GET_DATA_ERROR_LINE__(void)