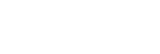 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
15 static int NNode, NElem;
21 static int set_ng_component(
void *src) {
22 Result->ng_component = *((
int *)src);
26 static int set_nn_component(
void *src) {
27 Result->nn_component = *((
int *)src);
31 static int set_ne_component(
void *src) {
32 Result->ne_component = *((
int *)src);
36 static int set_ng_dof(
void *src) {
39 if (
Result->ng_component <= 0)
return 0;
46 memcpy(
Result->ng_dof, src, size);
50 static int set_nn_dof(
void *src) {
53 if (
Result->nn_component <= 0)
return 0;
60 memcpy(
Result->nn_dof, src, size);
64 static int set_ne_dof(
void *src) {
67 if (
Result->ne_component <= 0)
return 0;
74 memcpy(
Result->ne_dof, src, size);
78 static int set_global_label(
void *src) {
81 if (
Result->ng_component <= 0)
return 0;
89 for (i = 0; i <
Result->ng_component; i++) {
97 static int set_node_label(
void *src) {
100 if (
Result->nn_component <= 0)
return 0;
108 for (i = 0; i <
Result->nn_component; i++) {
116 static int set_elem_label(
void *src) {
119 if (
Result->ne_component <= 0)
return 0;
127 for (i = 0; i <
Result->ne_component; i++) {
135 static int set_global_val_item(
void *src) {
139 if (
Result->ng_component <= 0)
return 0;
140 for (i = 0; i <
Result->ng_component; i++) {
143 size =
sizeof(*
Result->global_val_item) * n;
149 memcpy(
Result->global_val_item, src, size);
153 static int set_node_val_item(
void *src) {
157 if (
Result->nn_component <= 0)
return 0;
158 for (i = 0; i <
Result->nn_component; i++) {
161 size =
sizeof(*
Result->node_val_item) * n * NNode;
167 memcpy(
Result->node_val_item, src, size);
171 static int set_elem_val_item(
void *src) {
175 if (
Result->ne_component <= 0)
return 0;
176 for (i = 0; i <
Result->ne_component; i++) {
179 size =
sizeof(*
Result->elem_val_item) * n * NElem;
185 memcpy(
Result->elem_val_item, src, size);
195 static struct func_table {
201 {
"hecmwST_result_data",
"ng_component", set_ng_component},
202 {
"hecmwST_result_data",
"nn_component", set_nn_component},
203 {
"hecmwST_result_data",
"ne_component", set_ne_component},
204 {
"hecmwST_result_data",
"ng_dof", set_ng_dof},
205 {
"hecmwST_result_data",
"nn_dof", set_nn_dof},
206 {
"hecmwST_result_data",
"ne_dof", set_ne_dof},
207 {
"hecmwST_result_data",
"global_label", set_global_label},
208 {
"hecmwST_result_data",
"node_label", set_node_label},
209 {
"hecmwST_result_data",
"elem_label", set_elem_label},
210 {
"hecmwST_result_data",
"global_val_item", set_global_val_item},
211 {
"hecmwST_result_data",
"node_val_item", set_node_val_item},
212 {
"hecmwST_result_data",
"elem_val_item", set_elem_val_item},
215 static const int NFUNC =
sizeof(functions) /
sizeof(functions[0]);
217 static SetFunc get_set_func(
char *struct_name,
char *var_name) {
220 for (i = 0; i < NFUNC; i++) {
221 if (strcmp(functions[i].struct_name, struct_name) == 0 &&
222 strcmp(functions[i].var_name, var_name) == 0) {
223 return functions[i].set_func;
232 int n_node,
int n_elem) {
247 int *err,
int slen,
int vlen) {
257 "hecmw_result_copy_f2c_set_if(): 'result' has not initialized yet");
260 if (struct_name ==
NULL) {
262 "hecmw_result_copy_f2c_set_if(): 'sname' is NULL");
265 if (var_name ==
NULL) {
267 "hecmw_result_copy_f2c_set_if(): 'vname' is NULL");
279 func = get_set_func(sname, vname);
282 "hecmw_result_copy_f2c_set_if(): SetFunc not found");
294 int *err,
int slen,
int vlen) {
299 void *src,
int *err,
int slen,
305 void *src,
int *err,
int slen,
char * HECMW_result_get_comment(char *buff)
char * HECMW_result_get_header(char *buff)
#define HECMW_malloc(size)
void HECMW_RESULT_WRITE_ST_FINALIZE_IF(int *err)
void hecmw_result_write_st_finalize_if(int *err)
void hecmw_result_write_st_by_name_if(char *name_ID, int *err, int len)
void hecmw_result_write_st_finalize_if_(int *err)
void HECMW_RESULT_WRITE_ST_INIT_IF(int *err)
#define HECMW_calloc(nmemb, size)
void hecmw_result_write_st_init_if_(int *err)
int HECMW_result_write_ST_by_name(char *name_ID, struct hecmwST_result_data *result, int n_node, int n_elem, char *header, char *comment)
int HECMW_result_get_nnode(void)
void hecmw_result_write_st_by_name_if__(char *name_ID, int *err, int len)
void HECMW_RESULT_WRITE_ST_BY_NAME_IF(char *name_ID, int *err, int len)
void hecmw_result_write_st_init_if(int *err)
void hecmw_result_write_st_by_name_if_(char *name_ID, int *err, int len)
void hecmw_result_write_st_init_if__(int *err)
int HECMW_result_copy_f2c_init(struct hecmwST_result_data *result_data, int n_node, int n_elem)
void hecmw_result_write_st_finalize_if__(int *err)
void hecmw_result_copy_f2c_set_if_(char *struct_name, char *var_name, void *src, int *err, int slen, int vlen)
int HECMW_result_get_nelem(void)
char * HECMW_strcpy_f2c(const char *fstr, int flen)
void hecmw_result_copy_f2c_set_if(char *struct_name, char *var_name, void *src, int *err, int slen, int vlen)
char * HECMW_strcpy_f2c_r(const char *fstr, int flen, char *buf, int bufsize)
int HECMW_result_copy_f2c_finalize(void)
struct _result_struct Result
void HECMW_result_free(struct hecmwST_result_data *result)
int HECMW_set_error(int errorno, const char *fmt,...)
void hecmw_result_copy_f2c_set_if__(char *struct_name, char *var_name, void *src, int *err, int slen, int vlen)
void HECMW_RESULT_COPY_F2C_SET_IF(char *struct_name, char *var_name, void *src, int *err, int slen, int vlen)