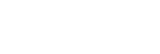 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
24 logical,
save :: NEED_ANALYSIS = .true.
25 type (sparse_matrix),
save :: spMAT
31 logical,
intent(in) :: is_sym
33 integer(kind=kint) :: spmat_type
34 integer(kind=kint) :: spmat_symtype
35 integer(kind=kint) :: i
44 if(hecmesh%PETOT.GT.1)
then
51 need_analysis = .true.
59 integer(kind=kint),
intent(out) :: istat
62 integer(kind=kint) :: phase_start
63 real(kind=
kreal) :: t1,t2
64 integer(kind=kint) :: mpc_method
69 if (mpc_method < 1 .or. 3 < mpc_method)
then
73 if (mpc_method /= 1)
then
74 write(*,*)
'ERROR: MPCMETHOD other than penalty is not available for DIRECTmkl solver', &
75 ' in contact analysis without elimination'
83 if (need_analysis)
then
89 if(
present(conmat))
then
100 if( hecmesh%PETOT .GT. 1 )
then
101 write(*,
'(A,f10.3)')
' [Cluster Pardiso]: Setup completed. time(sec)=',t2-t1
103 write(*,
'(A,f10.3)')
' [Pardiso]: Setup completed. time(sec)=',t2-t1
108 if (need_analysis)
then
110 need_analysis = .false.
114 if( hecmesh%PETOT.GT.1 )
then
119 deallocate(spmat%rhs)
subroutine, public hecmw_mat_dump_solution(hecMAT)
subroutine, public hecmw_clustermkl_wrapper(spMAT, phase_start, solution, istat)
subroutine, public sparse_matrix_set_type(spMAT, type, symtype)
real(kind=kreal) function hecmw_wtime()
subroutine, public hecmw_mat_set_mpc_method(hecMAT, mpc_method)
subroutine, public sparse_matrix_finalize(spMAT)
subroutine, public hecmw_mkl_wrapper(spMAT, phase_start, solx, istat)
integer(kind=kint), parameter, public sparse_matrix_type_csr
integer(kind=kint), parameter, public sparse_matrix_symtype_sym
integer(kind=4), parameter kreal
integer(kind=kint), parameter, public sparse_matrix_symtype_asym
integer(kind=kint), parameter, public sparse_matrix_type_coo
This module provides linear equation solver interface for Pardiso.
This module provides DOF based sparse matrix data structure (CSR and COO)
integer(kind=kint) function, public hecmw_mat_get_mpc_method(hecMAT)
subroutine, public hecmw_mat_dump(hecMAT, hecMESH)
subroutine, public hecmw_mat_ass_equation(hecMESH, hecMAT)
Structure for Lagrange multiplier-related part of stiffness matrix (Lagrange multiplier-related matri...
This module provides linear equation solver interface for Cluster Pardiso.
integer(kind=kint) function hecmw_comm_get_rank()
subroutine, public hecmw_mat_ass_equation_rhs(hecMESH, hecMAT)