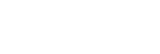 |
FrontISTR
5.7.0
Large-scale structural analysis program with finit element method
|
Go to the documentation of this file.
65 integer(kind=kint),
parameter ::
elastic = 110000
69 integer(kind=kint),
parameter ::
eplastic = 120000
71 integer(kind=kint),
parameter ::
neohooke = 130000
78 integer(kind=kint),
parameter ::
norton = 150000
84 integer(kind=kint),
parameter ::
d3 = -1
88 integer(kind=kint),
parameter ::
shell = 3
95 integer(kind=kint),
parameter ::
m_thick = 4
142 character(len=DICT_KEY_LENGTH) ::
mc_yield =
'YIELD'
152 integer(kind=kint) :: ortho
153 real(kind=kreal) :: ee
154 real(kind=kreal) :: pp
155 real(kind=kreal) :: ee2
156 real(kind=kreal) :: g12
157 real(kind=kreal) :: g23
158 real(kind=kreal) :: g31
159 real(kind=kreal) :: angle
160 real(kind=kreal) :: rho
161 real(kind=kreal) :: alpha
162 real(kind=kreal) :: alpha_over_mu
163 real(kind=kreal) :: weight
168 integer(kind=kint) :: nlgeom_flag
169 integer(kind=kint) :: mtype
170 integer(kind=kint) :: nfstatus
171 character(len=30) :: name
172 real(kind=kreal) :: variables(200)
173 integer(kind=kint) :: variables_i(200)
175 integer(kind=kint) :: totallyr
176 integer(kind=kint) :: cdsys_id
177 integer(kind=kint) :: n_table
178 real(kind=kreal),
pointer :: table(:)=>null()
179 type(dict_struct),
pointer :: dict
190 material%nfstatus = 0
192 material%variables = 0.d0
193 material%variables_i = 0
194 material%totallyr = 0
196 call dict_create( material%dict,
'INIT',
dict_null )
202 if(
associated(material%table) )
deallocate( material%table )
203 if(
associated(material%dict) )
call dict_destroy( material%dict )
208 integer,
intent(in) :: nm
230 integer,
intent(in) :: n
231 integer,
intent(in) :: m
232 real(kind=kreal),
intent(in) :: v
234 if( n>
size(
materials) .OR. m>100 )
return
240 integer,
intent(in) :: nfile
241 type(
tmaterial ),
intent(in) :: material
243 write( nfile, *)
"Material type:",material%mtype,material%nlgeom_flag
245 if( material%variables(i) /= 0.d0 )
write( nfile, *) i,material%variables(i)
247 if(
associated( material%table ) )
then
248 nt =
size(material%table)
249 write( nfile,* )
"--table--"
251 write(nfile,*) i,material%table(i)
259 integer,
intent(in) :: npos
260 integer,
intent(in) :: cnum
261 integer :: i, idum,cdum,dd
264 if( npos<=0 .or. npos>6)
return
265 if( cnum<100000 .or. cnum>999999 )
return
276 subroutine setdigit( npos, ival, mtype )
277 integer,
intent(in) :: npos
278 integer,
intent(in) :: ival
279 integer,
intent(inout) :: mtype
280 integer :: i, idum,cdum, cdum1, dd
282 if( npos<=0 .or. npos>6 )
return
283 if( ival<0 .or. ival>9 )
return
288 cdum1 = cdum1+ idum*dd
292 cdum1 = cdum1 + ival*dd
298 cdum1 = cdum1+ idum*dd
307 integer,
intent(in) :: mtype
311 if( itype/=1 )
return
313 if( itype/=1 .and. itype/=2 )
return
319 integer,
intent(in) :: mtype
323 if( itype/=1 )
return
325 if( itype/=2 )
return
331 integer,
intent(in) :: mtype
335 if( itype/=1 )
return
337 if( itype/=2 )
return
343 integer,
intent(in) :: mtype
352 integer,
intent(in) :: mtype
361 integer,
intent(in) :: mtype
370 integer,
intent(in) :: mtype
379 integer,
intent(in) :: mtype
387 subroutine ep2e( mtype )
388 integer,
intent(inout) :: mtype
395 integer,
intent(in) :: mtype
399 if( itype/=1 )
return
401 if( itype/=7 )
return
integer(kind=kint), parameter neohooke
integer(kind=kint), parameter m_plconst10
integer(kind=kint), parameter m_spring_axial
integer(kind=kint), parameter m_exapnsion
integer(kind=kint), parameter infinitesimal
character(len=dict_key_length) mc_viscoelastic
integer(kind=kint), parameter m_dashpot_a_ndoffset
character(len=dict_key_length) mc_yield
integer(kind=kint), parameter totallag
integer(kind=kint), parameter userhyperelastic
integer(kind=kint), parameter m_spring_dof
integer(kind=kint), parameter m_beam_angle1
integer(kind=kint), parameter m_density
integer function getnumofspring_aparam(material)
Get number of spring_a parameters.
character(len=dict_key_length) mc_isoelastic
character(len=dict_key_length) mc_incomp_newtonian
integer(kind=kint), parameter shell
integer(kind=kint), parameter axissymetric
integer function getnumofdashpot_aparam(material)
Get number of dashpot_a parameters.
subroutine printmaterial(nfile, material)
Print out the material properties.
integer(kind=kint), parameter m_beam_angle4
This module provides data structure table which would be dictionaried afterwards.
character(len=dict_key_length) mc_spring
logical function iskinematicharden(mtype)
If it is a kinematic hardening material?
subroutine initmaterial(material)
Initializer.
integer(kind=kint), parameter eplastic
integer(kind=kint), parameter mooneyrivlin_aniso
integer(kind=kint), parameter m_plconst2
integer function getelastictype(mtype)
Get elastic type.
character(len=dict_key_length) mc_dashpot
integer(kind=kint), parameter incomp_newtonian
integer(kind=kint), parameter m_beam_angle2
integer(kind=kint), parameter m_plconst6
integer(kind=kint), parameter m_plconst8
integer(kind=kint), parameter m_youngs
integer(kind=kint), parameter m_damping_rm
integer(kind=kint), parameter m_dashpot_dof
integer(kind=kint), parameter m_poisson
integer(kind=kint), parameter m_alpha_over_mu
integer(kind=kint), parameter m_plconst5
integer(kind=kint), parameter viscoelastic
integer(kind=kint), parameter m_spring_a_ndoffset
integer(kind=kint), parameter usermaterial
logical function iselastoplastic(mtype)
If it is an elastoplastic material?
integer(kind=kint), parameter m_plconst3
subroutine finalizematls()
Finalizer.
type(ttable), parameter dict_null
integer(kind=kint), parameter elastic
integer(kind=kint), parameter m_plconst7
integer(kind=kint), parameter m_beam_angle6
integer(kind=kint), parameter userelastic
integer(kind=kint), parameter m_kinehard
logical function iselastic(mtype)
If it is an elastic material?
type(tmaterial), dimension(:), allocatable materials
integer(kind=kint), parameter m_dashpot_d_ndoffset
character(len=dict_key_length) mc_orthoelastic
integer(kind=kint), parameter d3
subroutine initializematls(nm)
Initializer.
integer(kind=kint), parameter updatelag
integer(kind=kint), parameter m_thick
integer(kind=kint), parameter m_beam_angle5
subroutine modifymatl(n, m, v)
Set value of variable(m) of material n to v.
subroutine finalizematerial(material)
Finalizer.
integer(kind=kint), parameter m_beam_radius
integer(kind=kint), parameter m_plconst1
integer(kind=kint), parameter mn_orthoelastic
integer(kind=kint), parameter m_dashpot_axial
integer(kind=kint), parameter arrudaboyce
integer function getconnectortype(mtype)
Get type of connector.
character(len=dict_key_length) mc_orthoexp
subroutine ep2e(mtype)
Set material type of elastoplastic to elastic.
subroutine setdigit(npos, ival, mtype)
Modify material type.
integer(kind=kint), parameter m_spring_d_ndoffset
integer function getyieldfunction(mtype)
Get type of yield function.
Structure to manage all material related data.
integer(kind=kint), parameter m_plconst4
integer(kind=kint), parameter m_viscocity
integer(kind=kint), parameter m_beam_angle3
character(len=dict_key_length) mc_themoexp
integer(kind=kint), parameter planestrain
integer function getnumofdashpot_dparam(material)
Get number of dashpot_d parameters.
logical function isviscoelastic(mtype)
If it is an viscoelastic material?
integer(kind=kint), parameter m_plconst9
integer function fetchdigit(npos, cnum)
Fetch material type.
integer function getnumofspring_dparam(material)
Get number of spring_d parameters.
integer function gethardentype(mtype)
Get type of hardening.
This module summarizes all information of material properties.
integer(kind=kint), parameter m_damping_rk
This module provides data structure of dictionaried table list.
integer(kind=kint), parameter norton
integer(kind=kint), parameter planestress
integer(kind=kint), parameter mooneyrivlin
subroutine print_tabledata(dict, fname)
Print our the contents of a dictionary.
integer(kind=kint), parameter connector
logical function ishyperelastic(mtype)
If it is a hyperelastic material?
character(len=dict_key_length) mc_norton